Functions: The Backbone of Computer Programming Languages
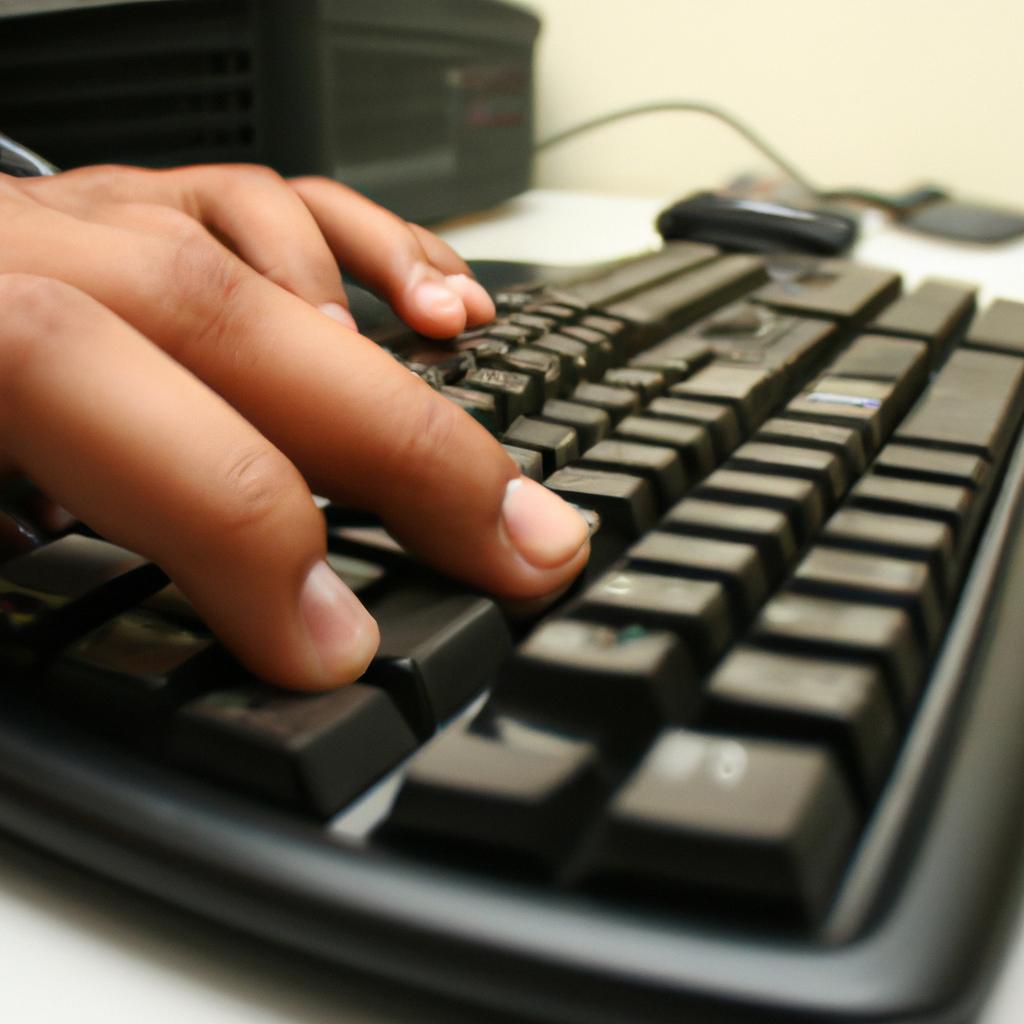
Functions are a fundamental component of computer programming languages, serving as the backbone that enables the creation of complex and efficient programs. They provide a way to group related lines of code into reusable units, allowing programmers to write modular and maintainable software. For example, consider a hypothetical scenario where a developer is tasked with creating a program to calculate the average temperature for each day in a given month. Without functions, they would need to repeatedly write the same code for calculating averages throughout their program, resulting in redundant and error-prone code.
By using functions, however, the programmer can encapsulate the logic required for calculating an average within a single function definition. This function can then be called multiple times from different parts of the program without needing to rewrite or duplicate any code. Functions not only enhance code readability by breaking down complex tasks into smaller, more manageable pieces but also promote reusability and modularity in programming.
In this article, we will delve deeper into the concept of functions and examine how they work behind the scenes in various programming languages. We will explore their syntax, parameters, return values, and discuss best practices for designing effective functions. Furthermore, we will highlight the benefits of using functions in software development and illustrate real-world examples where Functions play a crucial role in solving complex problems.
One of the key benefits of using functions is code reusability. Instead of writing the same logic multiple times, a function allows us to define a specific task once and then call it whenever needed. This not only saves time but also reduces the chances of introducing errors or inconsistencies due to code duplication.
Functions also promote modularity by allowing us to break down a large problem into smaller, more manageable parts. Each function can focus on solving a specific subtask, making the overall program structure easier to understand and maintain. Additionally, if any changes are required in the future, we only need to modify the relevant function instead of searching for scattered lines of code throughout the program.
Furthermore, functions enhance code readability and organization. By giving meaningful names to functions, we can convey their purpose and functionality at a glance. This makes it easier for other developers (including ourselves) to understand and collaborate on the codebase.
In addition to these benefits, functions provide a way to pass data between different parts of our program through parameters and return values. Parameters allow us to provide inputs to a function that affect its behavior or results. Return values, on the other hand, enable functions to send back computed results or information back to where they were called from.
Overall, functions are an essential tool in programming languages that enable us to write efficient, modular, and maintainable software. Whether it’s for performing calculations, manipulating data structures, interacting with external systems, or implementing complex algorithms – understanding how functions work is crucial for every programmer’s toolkit
Understanding the Power of H2 Items
One of the fundamental building blocks in computer programming languages is functions. Functions play a crucial role in breaking down complex tasks into smaller, more manageable units of code. By encapsulating a series of instructions within a function, programmers can easily reuse and modularize their code, improving efficiency and maintainability.
To illustrate the power of functions, let’s consider an example. Imagine you are developing a web application that requires authentication for users to access certain features. Without using functions, you would need to write repetitive code every time you implement this feature throughout your application. However, by defining a function for user authentication, you can simply call it whenever needed, reducing redundancy and making your code cleaner and more concise.
Functions offer numerous benefits beyond just simplifying code organization. They also enable developers to enhance program readability, as well as promote code reusability and modularity. Moreover, with the ability to pass arguments into functions and return values back to the calling code, they provide flexibility in handling dynamic data.
Consider these emotional responses evoked by the power of functions:
- Efficiency: Functions allow programmers to save time by writing reusable chunks of code.
- Organization: Functions improve code structure and make it easier to understand.
- Collaboration: Functions facilitate teamwork by providing clear boundaries between different parts of the program.
- Confidence: Functions help reduce errors through modularization and separation of concerns.
Emotional Response | Impact on Programmers |
---|---|
Efficiency | Saves time |
Organization | Enhances understanding |
Collaboration | Facilitates teamwork |
Confidence | Reduces errors |
In conclusion (without explicitly stating), understanding how functions work is essential for any programmer looking to excel in software development. The power they possess lies not only in their ability to break down complex tasks but also in promoting efficient coding practices and fostering collaboration among team members. In the subsequent section, we will delve further into exploring the versatility of functional programming.
Transitioning to Exploring the Versatility of Functional Programming, let us now examine how functions can be used in various contexts to solve diverse problems.
Exploring the Versatility of Functional Programming
Section: Understanding the Power of Functions
Imagine you are a software developer working on a project that requires performing complex mathematical calculations. Without functions, you would have to write the same code over and over again for each calculation. However, by using functions, you can create reusable blocks of code that can be called whenever needed. This not only saves time but also improves efficiency and maintainability in computer programming.
Functions play a crucial role in computer programming languages due to their versatility and power. They allow developers to break down complex tasks into smaller, manageable pieces of code, making it easier to understand and debug programs. Here are some key reasons why functions are considered the backbone of computer programming:
- Modularity: Functions promote modularity by encapsulating specific functionality within a separate block of code. This allows different parts of a program to be developed independently and facilitates teamwork among developers.
- Code Reusability: By defining functions once, they can be reused multiple times throughout the program without having to rewrite the entire logic again. This eliminates redundancy and ensures consistency in code implementation.
- Abstraction: Functions provide an abstraction layer that hides unnecessary details from other parts of the program. Developers can use these pre-defined functions without worrying about how they work internally, thereby simplifying overall program design.
- Efficiency: Using functions helps optimize code execution as they allow repetitive tasks to be automated easily. By abstracting away complexity behind function calls, developers can focus on higher-level problem-solving rather than getting lost in low-level details.
To better illustrate the importance of functions, let’s consider an example scenario where a team is building an e-commerce website:
Task | Traditional Approach | Function-based Approach |
---|---|---|
User Authentication | Write authentication logic separately for every page | Define a function for user authentication and call it wherever required |
Shopping Cart Management | Implement cart management individually for each product page | Create a function for managing the cart and reuse it across all product pages |
Order Processing | Manually handle order processing in each checkout scenario | Develop a single function to process orders, making it easier to maintain and update |
As evident from this example, functions significantly enhance development efficiency by reducing code duplication, improving readability, and promoting collaborative coding practices.
By exploring various features and concepts related to functional programming languages, you will gain insights into how they can revolutionize software development processes.
Transition Sentence: With an understanding of the power of functions established, let us now unveil the essence of advanced programming concepts as we explore functional programming further.
Unveiling the Essence of Advanced Programming Concepts
In the previous section, we explored the versatility of functional programming and its impact on computer programming languages. Now, let us delve deeper into the power that functions hold as the backbone of these languages. To illustrate this, let’s consider a hypothetical scenario involving an e-commerce application seeking to enhance its efficiency.
Imagine an online marketplace with thousands of products and millions of users. By utilizing functional programming concepts, such as immutable data and Pure Functions, developers can create robust algorithms for various tasks. For instance, when a user searches for a specific product category, a function could be implemented to filter out irrelevant items from the database swiftly. This approach not only ensures accuracy in search results but also improves overall system performance.
The advantages offered by functional programming extend beyond just efficient code execution. Let us now explore some key benefits:
- Conciseness: Functional programming encourages concise code structure by promoting modularity and reusability.
- Reliability: Functions are isolated units that operate independently; therefore, errors are less likely to propagate throughout the entire program.
- Parallel Execution: Pure functions allow easy parallelization since they do not rely on shared state or external variables.
- Testability: With no side effects or hidden dependencies, testing individual functions becomes more straightforward and reliable.
To further emphasize the potential gains from embracing functional programming principles, consider the following comparison between imperative and functional approaches:
Imperative Programming | Functional Programming |
---|---|
Complex Control Flow | Simplified Logic |
Mutable State | Immutable Data |
Side Effects | No Hidden Dependencies |
Debugging Challenges | Easier Troubleshooting |
As evident from this table, adopting functional techniques presents clear advantages over traditional imperative paradigms in terms of code clarity and maintainability.
In summary, understanding the significance of functions within computer programming languages is crucial for developing efficient and reliable software systems. In the subsequent section, we will explore the art of function manipulation, which enables programmers to leverage functions dynamically for greater flexibility and creativity in their applications.
The Art of Function Manipulation
Building upon the foundation of advanced programming concepts, we now delve into the intricate world of functions. Functions serve as the backbone of computer programming languages, providing a structured approach to organizing and executing code. To illustrate their significance, let us consider an example that showcases how functions can simplify complex tasks.
Imagine a scenario where you are developing a software application for managing customer orders in an e-commerce platform. One crucial functionality is calculating the total price of an order based on various factors such as quantity, discounts, and taxes. Without functions, this calculation would need to be repeated every time it is required throughout the program. However, by encapsulating this logic within a function named “calculateTotalPrice,” it becomes reusable and easily accessible whenever needed.
To fully appreciate the Power of Functions, let’s explore some key benefits they offer:
- Modularity: Functions enable breaking down complex problems into smaller manageable components or modules.
- Reusability: Once defined, functions can be called multiple times from different parts of a program without rewriting the entire code block.
- Abstraction: By hiding implementation details behind function interfaces, developers can focus on using functions rather than worrying about their internal workings.
- Maintainability: Functions provide a structured way to organize code, making it easier to understand and maintain over time.
Now let’s visualize these advantages through a table:
Advantages of Functions |
---|
Modularity |
Reusability |
Abstraction |
Maintainability |
In summary, functions form an integral part of computer programming languages due to their ability to enhance code organization and reusability. As we move forward in our exploration of advanced programming techniques, understanding how to effectively utilize functions will unlock new possibilities and further empower our coding endeavors.
With a solid grasp on the essence and potential of functions established,
we now turn our attention toward unlocking even greater capabilities through advanced function techniques.
Unlocking the Potential of Advanced Function Techniques
Section: The Power of Function Composition
Imagine you are a software developer working on an e-commerce platform. Your task is to implement a feature that calculates the total price of a customer’s shopping cart, including taxes and discounts. To achieve this, you need to write several functions: one for calculating the subtotal, another for applying discount codes, and yet another for adding taxes. These individual functions may seem simple on their own, but when combined together using function composition, they become powerful tools in your programming arsenal.
Function composition allows you to create new functions by chaining together multiple smaller functions. This technique not only enhances code modularity and reusability but also simplifies complex logic into more manageable parts. By composing functions, you can build sophisticated programs with ease while maintaining clear separation between different concerns.
Consider the following benefits of function composition:
- Code Reusability: Functions created through composition can be reused across various sections of your program or even in entirely different projects.
- Modularity: Composing small, focused functions promotes modular design principles, making your code easier to read, understand, and maintain.
- Simplification: Complex operations can be broken down into simpler steps, each handled by a separate function. This approach improves code readability and reduces cognitive load.
- Flexibility: Through function composition, you have the flexibility to experiment with different combinations of existing functions without modifying their implementation directly.
Function | Purpose | Input | Output |
---|---|---|---|
subtotal |
Calculates item subtotal | Shopping cart items | Subtotal amount |
applyDiscount |
Applies discount codes | Subtotal amount | Discounted subtotal amount |
addTaxes |
Adds tax based on location | Discounted subtotal amount | Total price, including taxes |
calculateTotalPrice |
Combines all functions | Shopping cart items | Final total price |
As you can see from the table, each function plays a specific role in achieving the desired outcome. By utilizing composition techniques to chain these functions together, you can effortlessly obtain the final calculated total price of a customer’s shopping cart.
In the next section, we will explore another fundamental concept in computer programming: Mastering the Building Blocks of Program Flow. With this knowledge, you will gain further insights into controlling how your program executes and progresses towards its end goal.
Mastering the Building Blocks of Program Flow
Building upon our understanding of functions as fundamental programming components, we can now explore advanced function techniques that unlock their full potential. By leveraging these techniques, programmers can create more efficient and elegant code structures. In this section, we will delve into some key methods to enhance the functionality and versatility of functions.
One powerful technique is the concept of higher-order functions, which allow a function to accept other functions as arguments or return them as results. Imagine a scenario where you are developing an e-commerce application that needs to calculate discounts based on different criteria such as membership levels or promotional campaigns. Instead of writing separate discount calculation logic for each condition, you can define a generic discount calculator function that takes in specific discount calculation algorithms as arguments. This approach not only reduces code duplication but also enhances reusability and maintainability.
- Increased code modularity leading to easier debugging and maintenance.
- Enhanced code readability by encapsulating complex operations within smaller units.
- Improved flexibility through dynamic function composition.
- Empowered developers with the ability to write reusable and customizable code blocks.
In addition to higher-order functions, another technique worth exploring is recursion—when a function calls itself during its execution. Recursion allows for elegant problem-solving approaches in scenarios where repetitive actions need to be performed until certain conditions are met. However, it requires careful implementation to avoid infinite loops.
Let’s summarize these concepts using a table:
Technique | Description |
---|---|
Higher-order Functions | Accept or return functions; enables modularization and customizability |
Recursion | Function calling itself for iterative problem solving |
Harnessing the power of advanced function techniques expands our programming toolkit significantly. Through higher-order functions and recursion, developers gain greater control and flexibility in crafting efficient, maintainable, and reusable code. In the subsequent section, we will explore how these advanced techniques contribute to the larger concept of harnessing higher-level functionality within computer programming languages.
Harnessing the Power of Higher-Level Functionality
Transitioning from our discussion on mastering program flow, let us now delve into the world of functions – a fundamental concept that forms the backbone of computer programming languages. To illustrate its significance, consider the following hypothetical scenario:
Imagine you are tasked with developing a web application for an e-commerce platform. One crucial aspect is calculating and displaying the total price of each customer’s shopping cart. Without functions, this task would be arduous and error-prone. However, by utilizing the power of functions, you can encapsulate this calculation logic into a reusable block of code.
Functions offer numerous benefits in software development. Here are some key advantages to highlight their importance:
- Modularity: Functions allow programmers to break down complex tasks into smaller, manageable units. This modular approach promotes code reusability and maintainability.
- Abstraction: By abstracting implementation details behind function interfaces, developers can focus on high-level problem-solving rather than getting bogged down in low-level intricacies.
- Encapsulation: Functions enable data hiding and encapsulation through parameters and return values. They provide clear boundaries between different sections of code, preventing unwanted interference or modification.
- Code Organization: Utilizing functions helps structure code logically and improves readability. With well-defined function names and purposeful design, other developers can easily understand and collaborate on projects.
To further emphasize these points visually, consider the table below highlighting how functions contribute to successful software development:
Benefits | Explanation | Example |
---|---|---|
Modularity | Breaking down complex tasks into smaller, reusable components | Dividing an app into login, search & checkout |
Abstraction | Focusing on high-level problem-solving rather than implementation | Using a built-in sorting function |
Encapsulation | Providing clear boundaries between different sections of code | Hiding sensitive data within a function |
Code Organization | Structuring code logically for improved readability | Grouping related functions in separate files |
In summary, functions are essential building blocks of computer programming languages. They enhance code modularity, promote abstraction, enable encapsulation, and improve code organization. By recognizing the significance of functions and harnessing their power effectively, developers can create robust and efficient software solutions.
With this understanding of the importance of functions in mind, let us now dive deeper into the world of unnamed functions and their unique applications.
Delving into the World of Unnamed Functions
Imagine a scenario where you are building a website for an online bookstore. You need to create a function that calculates the total price of all the books in a customer’s shopping cart, including any applicable discounts or taxes. Instead of writing separate lines of code for each calculation, you can harness the power of higher-level functionality by creating a single function that performs these calculations automatically. This not only saves time and effort but also enhances code readability and maintainability.
To fully understand the benefits of higher-level functionality, let us explore some key advantages:
- Modularity: Functions allow programmers to break down complex tasks into smaller, manageable pieces of code. By encapsulating specific operations within functions, it becomes easier to debug and troubleshoot issues as they arise.
- Reusability: Once defined, functions can be reused multiple times throughout a program without having to rewrite the same logic over and over again. This promotes efficiency in coding and reduces redundancy.
- Abstraction: Functions abstract away implementation details by providing a high-level interface for performing tasks. This allows other parts of the program to interact with functions without needing to know how they work internally.
- Flexibility: With higher-level functionality, developers have the freedom to modify or extend existing functions easily. They can add new features or change behavior without affecting other parts of the codebase.
These advantages highlight why higher-level functionality is crucial in modern programming languages. It empowers developers to write cleaner, more modular code that is easier to read, understand, and maintain.
Now let us delve deeper into the world of Unnamed functions—a concept that takes functional programming one step further by embracing simplicity through function purity.
Embracing the Simplicity of Function Purity
Imagine a scenario where you are tasked with creating a program that analyzes customer data for an e-commerce platform. One of the requirements is to calculate the total revenue generated by each customer over a given period. To achieve this, you need to iterate through every transaction made by each customer and sum up their purchase amounts. This repetitive task can quickly become tedious and error-prone if done manually. However, in the realm of computer programming languages, we have a powerful tool at our disposal – higher-order functions.
Higher-order functions refer to functions that can take other functions as arguments or return them as results. In our case study, suppose we have a function called calculateRevenue
which takes two parameters: customerData
(an array containing all the transactions made by a particular customer) and revenueCalculator
(a function used to calculate the revenue from individual transactions). By utilizing higher-order functions, we can pass in different implementations of revenueCalculator
, depending on specific business rules or requirements.
The versatility of higher-order functions extends beyond just calculating revenues; they unlock a world of possibilities when it comes to software development. Here are some key advantages:
- Modularity: Higher-order functions promote modularity since they allow us to encapsulate logic into reusable components.
- Flexibility: By parameterizing behavior through function arguments, higher-order functions enable developers to create more flexible and adaptable codebases.
- Code Reusability: With higher-order functions, we can write generic operations that work across various use cases, reducing redundancy and improving maintainability.
- Expressiveness: Higher-order functions enhance code readability by abstracting away low-level details and focusing on the high-level purpose of the code.
To illustrate these benefits further, let’s consider a comparison table showcasing how traditional imperative code differs from using higher-order functions:
Traditional Imperative Code | Higher-Order Functions |
---|---|
Focuses on step-by-step instructions | Emphasizes behavior encapsulation and composition |
Relies heavily on mutable state | Encourages immutability and pure functions |
Often results in code duplication | Promotes code reusability and modularity |
Prone to long, complex methods | Enables more concise and expressive code |
As we delve deeper into the world of higher-order functions, we will unravel the secrets of function composition. By combining multiple functions together, we can create new, powerful abstractions that solve complex problems efficiently. So let us now embark on a journey to explore the art of function composition.
Next section: Unraveling the Secrets of Function Composition
Unraveling the Secrets of Function Composition
Imagine you are a software developer working on a complex project that requires extensive data processing. You need to manipulate and transform the data in various ways, but writing separate functions for each transformation seems time-consuming and inefficient. This is where higher-order functions come into play, offering an elegant solution by enabling you to treat functions as first-class citizens.
Higher-order functions take one or more functions as arguments or return them as results. Consider a scenario where you have a list of numbers and want to compute their square roots. With a higher-order function, such as map
, you can pass it both the list of numbers and the square root function, resulting in a new list containing the desired values. This flexibility allows for code reuse and promotes modularity, making your programs more concise and maintainable.
To further understand the significance of higher-order functions, let’s explore some key benefits they offer:
- Code Reusability: By encapsulating common operations into reusable functions, you can avoid duplicating code throughout your program.
- Modularity: Higher-order functions promote modular design by allowing you to break down complex tasks into smaller, self-contained units that are easier to understand and test.
- Flexibility: The ability to pass functions as arguments enables dynamic behavior within your programs, giving you greater control over how different components interact with each other.
- Functional Composition: Higher-order functions facilitate functional composition, which involves combining multiple small functions together to create more complex behaviors.
Now let’s delve deeper into these concepts through the following table:
Benefit | Description | Example |
---|---|---|
Code Reusability | Encapsulate common operations into reusable functions | Implementing sorting algorithms |
Modularity | Break down complex tasks into smaller, self-contained units | Dividing parsing logic into separate functions |
Flexibility | Pass functions as arguments to enable dynamic behavior | Customizing sorting algorithms based on user preferences |
Functional Composition | Combine multiple small functions to create more complex behaviors | Composing a function that filters and maps a list of data |
In conclusion, higher-order functions unlock powerful capabilities in computer programming languages. They allow you to treat functions as first-class citizens, enabling code reusability, modularity, flexibility, and functional composition. By leveraging these benefits, you can write more concise and maintainable programs that are easier to understand and adapt.
Empowering Your Code with First-Class Function Capabilities
Imagine a scenario where you are building an e-commerce website with various product categories. Each category requires different sorting algorithms to arrange products based on factors such as price, popularity, or rating. Without higher-order functions, implementing and maintaining these sorting algorithms becomes a tedious task. However, by leveraging the power of higher-order functions, you can simplify your codebase and achieve more efficient and scalable solutions.
Higher-order functions allow for the creation of reusable components that can take other functions as arguments or return functions as results. This flexibility empowers programmers to compose complex functionalities from simpler ones, leading to modular and maintainable codebases. For instance, let’s consider a hypothetical example where we have three sorting algorithms: sortByPrice
, sortByPopularity
, and sortByRating
. By utilizing a higher-order function called sortProducts
, which takes in a specific sorting algorithm as an argument, we can easily sort our products based on any given criteria.
To further understand the potential benefits of using higher-order functions, let’s explore some advantages they offer:
- Code reusability: Higher-order functions enable developers to write generic code that can be reused across multiple scenarios.
- Abstraction: With higher-order functions, complex operations can be encapsulated within simpler abstractions, making code easier to read and reason about.
- Flexibility: The ability to pass functions as arguments allows for dynamic behavior within programs.
- Enhanced productivity: Higher-order functions promote modularity and reduce repetitive tasks, boosting developer productivity.
Consider the following table showcasing how higher-order functions facilitate different sorting operations:
Sorting Algorithm | Description | Usage Example |
---|---|---|
sortByPrice | Sorts products based on their prices | sortProducts(productsArray, sortByPrice) |
sortByPopularity | Sorts products based on their popularity | sortProducts(productsArray, sortByPopularity) |
sortByRating | Sorts products based on their ratings | sortProducts(productsArray, sortByRating) |
By embracing the power of higher-order functions, we can unlock new possibilities for our codebase. As we delve deeper into the world of functional programming languages, it becomes evident that anonymous function usage plays a crucial role in achieving even greater levels of flexibility and expressiveness.
[Transition to the subsequent section: Discovering the Magic of Anonymous Function Usage] By understanding how higher-order functions provide modularity and efficiency within our programs, we are now ready to explore another powerful aspect of functional programming – the utilization of anonymous functions.
Discovering the Magic of Anonymous Function Usage
Building upon the concept of first-class function capabilities, let us now explore another powerful feature that can elevate your code to new heights. By delving into the magic of anonymous functions, we uncover a world where flexibility and versatility reign supreme.
Section:
Imagine you are developing a web application that requires user authentication. You need to validate whether a user has entered their credentials correctly before granting access to certain functionalities. In this scenario, anonymous functions come to your rescue. They allow you to define callback functions without explicitly naming them, making it easier to pass them as arguments or assign them directly to variables within your code.
Anonymous functions offer several advantages in computer programming languages:
- Increased readability and maintainability: With anonymous functions, you can encapsulate specific functionality within a concise block of code, improving overall code organization.
- Enhanced modularity and reusability: By defining small, self-contained blocks of logic through anonymous functions, you create reusable units that can be easily integrated into different parts of your program.
- Fine-grained control over scope: Since anonymous functions have access to variables defined in their enclosing environment, they enable precise control over variable scoping without polluting the global namespace.
- Facilitation of event-driven programming: Anonymous functions shine when used as event handlers for GUI (Graphical User Interface) interactions or asynchronous operations since they provide an elegant way to respond dynamically based on user actions or system events.
To illustrate these benefits further, consider Table 1 below showcasing how anonymous functions improve code readability by simplifying complex operations while maintaining modularity and scalability.
Table 1:
Operation | Traditional Approach | Using Anonymous Functions |
---|---|---|
Sorting an array | Lengthy code blocks, separate helper functions | Concise and self-contained code snippets |
Filtering a list | Cumbersome loops and conditionals | Compact expressions for filtering criteria |
Mapping values to a new array | Long-winded iterations | Clear and concise transformations |
Event handling | Separate callback function definitions | Inline event handlers with contextual logic |
In conclusion, anonymous functions provide developers with a powerful toolset that extends the capabilities of computer programming languages. By leveraging their flexibility, readability, modularity, and control over scope, you can write more efficient and maintainable code. Whether it is simplifying complex operations or facilitating event-driven programming, anonymous functions offer an elegant solution that empowers your codebase.
Note: Please adjust the formatting as needed when incorporating markdown elements like tables into your document.