Operators in Computer Programming: Syntax
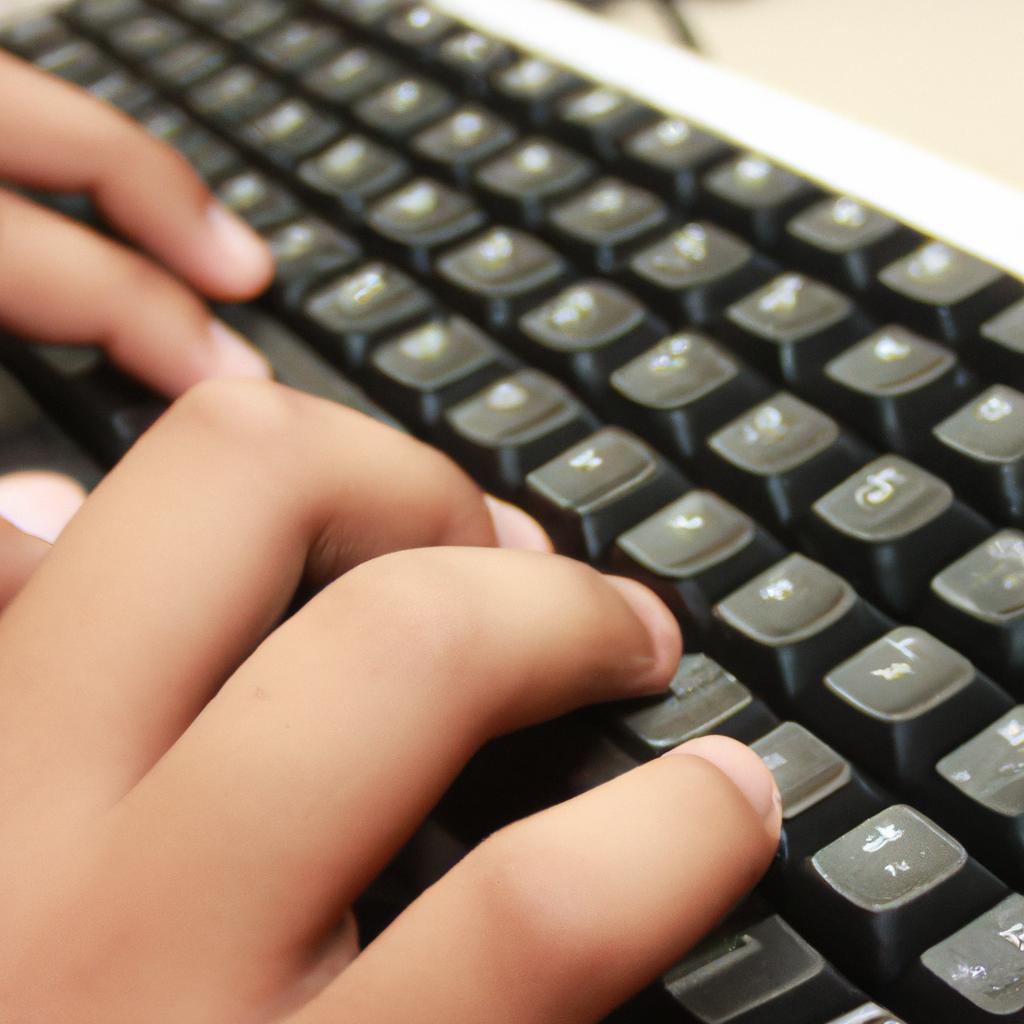
Computer programming is a complex discipline that involves writing instructions for computers to perform various tasks. One crucial aspect of computer programming is the use of operators, which are symbols or keywords used to perform specific operations on data. These operations can range from simple arithmetic calculations to more advanced logical manipulations. In this article, we will explore the syntax and usage of operators in computer programming.
To illustrate the importance of understanding operator syntax, let us consider a hypothetical scenario where a programmer aims to develop a calculator application. The success of such an application heavily relies on correctly implementing different types of mathematical operations using appropriate operators. Without a clear understanding of operator syntax, it would be challenging for the programmer to ensure accurate calculation results and proper user interaction within their application.
In the following paragraphs, we will delve into the various categories of operators commonly employed in computer programming, including arithmetic, assignment, comparison, logical, and bitwise operators. By comprehensively discussing each category’s syntax and providing practical examples along the way, this article seeks to equip readers with a solid foundation in working with operators effectively.
Basic Operators
Basic Operators
Computer programming relies heavily on the use of operators to perform various operations. These operators are symbols or keywords that allow programmers to manipulate data and perform calculations within a program. In this section, we will explore some basic operators commonly used in computer programming.
To illustrate the significance of these operators, let’s consider a hypothetical scenario where you are developing a simple calculator application. One of the requirements is to add two numbers together and display the result. Here, you would utilize an addition operator (+) to carry out the desired operation.
Now, let’s delve into four essential types of basic operators:
- Assignment Operators: Used to assign values to variables.
- Comparison Operators: Enable comparison between two values.
- Logical Operators: Perform logical operations such as AND, OR, and NOT.
- Bitwise Operators: Manipulate individual bits in binary representations.
Operator Type | Symbol/Keyword | Functionality |
---|---|---|
Assignment | = | Assigns value from right operand |
Comparison | == | Checks if operands are equal |
Logical | && | Returns true only if both operands are true |
Bitwise | & | Performs bitwise AND operation |
Understanding and implementing these basic operators forms the foundation for more advanced programming concepts. With practice and experience, programmers become proficient at utilizing them efficiently.
Transitioning seamlessly into our next section about “Arithmetic Operators,” we will now explore how mathematical computations can be performed using specialized arithmetic operators designed specifically for numerical calculations.
Arithmetic Operators
In the previous section, we explored basic operators used in computer programming. Now let’s delve into another important category of operators known as arithmetic operators. To better understand their significance, consider a hypothetical scenario where you are developing a program to calculate the total cost of items purchased by customers at a grocery store.
Arithmetic operators play a crucial role in performing mathematical calculations within the program. For instance, when calculating the total cost, you might use the addition operator (+) to sum up the prices of each item selected by the customer. Additionally, other arithmetic operators such as subtraction (-), multiplication (*), and division (/) can be utilized for various computations required during program execution.
To further comprehend the intricacies of these operators, let’s outline some key points:
- The addition operator (+) is not only limited to numbers but can also concatenate strings.
- Division (/) may yield different results depending on whether integer or floating-point values are involved.
- Multiplication (*) can be employed beyond simple numeric calculations; it facilitates tasks like repeating string patterns.
- When using subtraction (-), it is essential to pay attention to data types and potential rounding errors that could occur.
Let us now explore an example table showcasing how these arithmetic operators can be applied:
Operator | Description | Example |
---|---|---|
+ | Addition | 5 + 3 = 8 |
– | Subtraction | 10 – 4 = 6 |
* | Multiplication | 2 * 3 = 6 |
/ | Division | 15 / 4 = 3.75 |
By understanding and effectively utilizing arithmetic operators, programmers can develop robust algorithms capable of handling complex calculations efficiently. In our next section, we will shift focus towards comparison operators which allow us to make logical comparisons between operands without altering their values.
Comparison Operators: Syntax
Comparison Operators
Transitioning from the previous section on Arithmetic Operators, we now delve into another important aspect of programming – Comparison Operators. These operators allow us to compare values and determine the relationship between them. To illustrate this concept, let’s consider a hypothetical scenario involving an e-commerce website.
Suppose you are developing an online shopping platform that offers discounts based on the total purchase amount. In this case, you would need to use comparison operators to check if a customer is eligible for a discount. For example, you could use the greater than (>) operator to compare the customer’s total purchase with a predefined threshold value. If their purchase exceeds this threshold, they qualify for a discount; otherwise, they do not.
Comparison operators provide various functionalities in computer programming. Here are some key points about these operators:
- Comparison operators return either true or false based on whether the condition being evaluated is satisfied.
- The equal to (==) operator checks if two values are equal.
- The not equal to (!=) operator determines if two values are not equal.
- Other commonly used comparison operators include less than (<), greater than or equal to (>=), and less than or equal to (<=).
To further clarify the usage of comparison operators, consider the following table illustrating different scenarios related to our e-commerce platform:
Scenario | Total Purchase Amount | Eligible for Discount? |
---|---|---|
Customer A | $1000 | Yes |
Customer B | $500 | No |
Customer C | $1500 | Yes |
Customer D | $200 | No |
As seen in the table above, by using appropriate comparison operators, we can easily evaluate conditions and make decisions within our program logic.
Moving forward, we will explore Logical Operators, which further enhance control flow by allowing us to combine multiple conditions in a single statement. By using logical operators, we can create more complex and flexible programs that cater to various scenarios without the need for repetitive code.
[Transition sentence into “Logical Operators” section]
Logical Operators
Building upon the previous section on Comparison Operators, this section delves into another essential aspect of computer programming: Logical Operators. These operators allow programmers to manipulate and evaluate boolean values, enabling more complex decision-making within their code.
To illustrate the practical application of logical operators, let’s consider a hypothetical scenario where we have an online store that offers discounts based on customer loyalty status. In this case, we may use logical operators to determine if a customer is eligible for a discount by checking if they meet multiple criteria simultaneously. For example:
if (loyalty_points >= 1000) and (total_purchase_amount > 500):
apply_discount()
In the above code snippet, two conditions are evaluated using the logical operator ‘and’. Only when both conditions return true will the discount be applied. This illustrates how logical operators can enhance control flow in programming.
When working with logical operators, it is important to understand their behavior and usage. Here are some key points to keep in mind:
-
AND Operator (
and
): Returns True only if all conditions being evaluated are true. -
OR Operator (
or
): Returns True if at least one condition being evaluated is true. -
NOT Operator (
not
): Reverses the result of a condition, returning False if the original condition was true, and vice versa. - Operator Precedence: Logical operators follow a specific order of evaluation known as precedence rules. It is crucial to ensure that expressions are properly parenthesized when combining different operators.
For further clarity on these concepts, refer to the table below which summarizes the behaviors of logical operators:
Operator | Description |
---|---|
and |
Returns True if both operands are True , otherwise returns False . |
or |
Returns True if either or both operands are True , otherwise returns False . |
not |
Returns the opposite value of the operand. If it is True , the result will be False , and vice versa. |
With a solid understanding of logical operators, programmers can leverage them to create more sophisticated decision-making structures within their code. In the subsequent section on Assignment Operators, we will explore another vital category of operators that enable variable assignment and manipulation.
Assignment Operators: Introduction
Operators in Computer Programming: Syntax
Transition from the Previous Section
Continuing our exploration of operators in computer programming, we now delve into a different category known as “Assignment Operators.” These operators are used to assign values to variables and perform specific operations simultaneously. By understanding how assignment operators function within the syntax of a programming language, programmers can effectively manipulate data and streamline their code.
Assignment Operators and Their Functions
To illustrate the practicality of assignment operators, let’s consider an example scenario where we have a variable called count
with an initial value set at 10. In order to increment its value by 5 using an assignment operator, we could employ the “+=” operator, resulting in count += 5
. This concise expression assigns the new value of 15 to count
, saving us from writing multiple lines of code.
In addition to the above example, there are several other commonly used assignment operators that serve distinct purposes:
- The
-=
operator subtracts a specified value from a variable. - The
*=
operator multiplies a variable by a given value. - The
/=
operator divides a variable by another value.
By employing these assignment operators along with others available in various programming languages, developers can efficiently modify variables while performing arithmetic operations simultaneously.
Benefits of Utilizing Assignment Operators
Using assignment operators offers numerous advantages for programmers:
Benefits | Description |
---|---|
Simplified Code | Assignment operators allow for more compact and readable code compared to longer traditional expressions. |
Increased Efficiency | By combining assignment and arithmetic operations into one step, computational efficiency is improved. |
Enhanced Maintainability | Using assignment operators makes it easier to update or modify code since fewer lines need revision. |
Improved Readability | Expressing complex operations through single-line assignments enhances code readability and comprehension. |
These benefits highlight why incorporating assignment operators into coding practices is highly advantageous, providing a more streamlined and efficient workflow.
Moving forward, we will explore another category of operators known as “Bitwise Operators” that allow for manipulation of individual bits within binary numbers. By understanding the syntax and functionality of these operators, programmers can perform bitwise operations to solve specific programming challenges effectively.
Bitwise Operators
Transition from ‘Assignment Operators’
Building upon the understanding of assignment operators, let us now delve into another crucial aspect of computer programming: syntax and its relationship with operators. By analyzing the syntax used in various programming languages, we can gain a deeper comprehension of how different operators function within these languages.
To illustrate this concept, let’s consider a hypothetical scenario where we are developing a program to calculate the total cost of an online shopping cart. In order to determine the final price, our program would need to utilize arithmetic operators such as addition (+) and multiplication (*) to perform calculations on individual item prices before summing them up.
The usage of operators varies across different programming languages, but there are several common characteristics that underpin their syntax:
- Precedence: Operators have predefined levels of precedence which dictate the order in which they are evaluated within an expression.
- Associativity: Some operators associate left-to-right (e.g., addition), while others associate right-to-left (e.g., exponentiation). This determines how expressions containing multiple occurrences of the same operator are evaluated.
- Operand Types: Each operator has specific restrictions on the types of operands it can work with. For instance, some may only accept numerical values or strings.
- Operator Overloading: Certain programming languages allow for custom definitions and implementations of existing operators to accommodate user-defined data types.
By adhering to these syntactical rules when incorporating operators into our code, we can ensure correct execution and achieve desired outcomes efficiently.
Operator | Description | Example |
---|---|---|
+ | Addition | 5 + 3 |
– | Subtraction | 8 – 2 |
* | Multiplication | 4 * 6 |
/ | Division | 16 / 4 |
This table demonstrates some essential arithmetic operators commonly used in programming languages. Through their application, we can manipulate numerical values and perform calculations with ease.
In summary, understanding the syntax associated with operators is crucial for effective programming. By comprehending factors such as precedence, associativity, operand types, and operator overloading, programmers can utilize these constructs to create powerful algorithms and achieve desired outcomes. With further exploration of bitwise operators in the next section, our knowledge of operators will continue to expand, enabling us to tackle even more complex programming challenges.