Lambda Functions: The Power of Functions in Computer Programming Languages
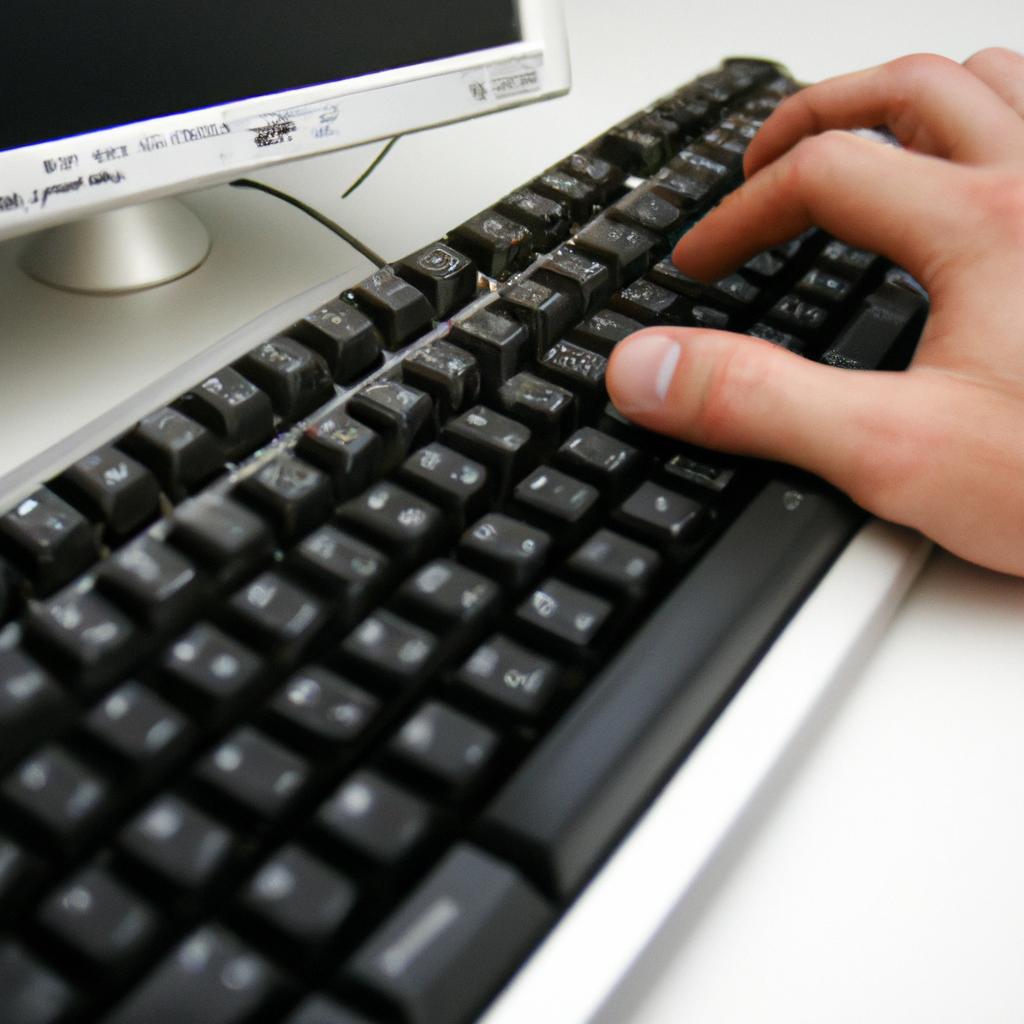
Lambda functions, also known as anonymous functions or function literals, are a powerful concept in computer programming languages. They provide a concise and flexible way to define and use functions within code. Lambda functions have gained significant popularity in recent years due to their ability to simplify complex tasks and enhance code readability. To illustrate the impact of lambda functions, consider the example of a web application that requires sorting a list of user names based on their length. By using lambda functions, developers can efficiently implement this functionality without writing lengthy and repetitive code.
In computer programming languages, traditional named functions serve as reusable blocks of code that perform specific tasks when called upon. However, lambda functions offer an alternative approach by allowing programmers to create temporary or one-time-use functions without explicitly naming them. This feature makes lambda functions particularly useful for situations where defining a separate function is unnecessary or time-consuming. The flexibility provided by these anonymous functions allows developers to write more expressive and efficient code while reducing complexity and increasing maintainability. Additionally, many modern programming languages support higher-order functions, which means lambda functions can be passed as arguments to other functions or stored in variables for later use, further extending their versatility and power in solving computational problems.
What are Lambda Functions?
Lambda functions, also known as anonymous functions or function literals, are a powerful concept in computer programming languages. These functions allow developers to write concise and efficient code by creating small, self-contained units of functionality that can be used without explicit declaration.
To illustrate the significance of lambda functions, let’s consider a real-world scenario where we want to sort a list of names alphabetically. Traditionally, one would need to define a separate sorting function with several lines of code. However, using lambda functions, this task becomes much simpler. For example:
names = ["Alice", "Bob", "Charlie"]
sorted_names = sorted(names, key=lambda x: x.lower())
In this case, the lambda function acts as an inline sorting criterion for the sorted()
function. By specifying key=lambda x: x.lower()
, we instruct Python to sort the names based on their lowercase values. This concise syntax allows us to achieve our goal in just one line.
The benefits of using lambda functions extend beyond brevity and ease-of-use. They provide programmers with added flexibility and expressiveness in their coding practices. Here are some reasons why lambda functions have become increasingly popular:
- Conciseness: Lambda functions allow for shorter and more readable code by eliminating the need for defining separate named functions.
- Flexibility: With lambda functions, developers can create ad-hoc functionality tailored specifically for a particular use case without cluttering their codebase.
- Higher-order operations: Lambda functions enable higher-order operations like mapping and filtering elements from collections effortlessly.
Pros | Cons | Neutral |
---|---|---|
Concise | Lack of reusability | Easier debugging |
Flexible | Limited scope | Improved readability |
Higher-order operations | Less explicit | Simplified code maintenance |
In conclusion, lambda functions offer a concise and flexible way to write small, functional units of code in computer programming languages. Their ability to enhance readability and facilitate higher-order operations makes them an indispensable tool for developers. In the subsequent section, we will explore the benefits of incorporating lambda functions into your coding practices.
Benefits of Lambda Functions
[Transition sentence] Now that we have understood what lambda functions are and their significance in computer programming languages, let’s delve deeper into the various advantages they bring to software development processes.
Benefits of Lambda Functions
Section H2: Overview of Lambda Functions
In the previous section, we explored the concept of lambda functions and their significance in computer programming languages. Now, let’s delve deeper into the benefits that these functions offer to programmers.
Imagine a scenario where you are working on a project with tight deadlines. You need to sort a large dataset based on multiple criteria quickly and efficiently. This is where lambda functions come to the rescue! By using lambda functions, you can create concise and powerful sorting algorithms without having to write lengthy code or define separate named functions for each criterion.
To further understand the advantages of lambda functions, consider the following:
- Expressiveness: Lambda functions allow developers to express complex operations concisely within a single line of code.
- Flexibility: With lambda functions, programmers have more control over defining anonymous functions tailored specifically for certain tasks.
- Readability: The compactness of lambda expressions enhances code readability by eliminating unnecessary function definitions.
- Efficiency: Due to their lightweight nature, lambda functions contribute to optimized performance when executing repetitive tasks.
Advantages of Lambda Functions |
---|
1. Concise syntax allows for faster development. |
2. Increased flexibility enables customization as per requirements. |
3. Enhanced readability leads to better understanding and maintenance of codebase. |
4. Improved efficiency results in faster execution time for computationally intensive operations. |
Considering these benefits, it is evident why many modern programming languages provide support for lambda functions. In the upcoming section, we will explore the syntax used to define and utilize these powerful constructs seamlessly.
Transitioning smoothly into the subsequent section about “Syntax of Lambda Functions,” let us now examine how these expressive tools are implemented within various programming languages’ frameworks and libraries.
Syntax of Lambda Functions
Now that we have explored the concept of lambda functions, let us delve deeper into their syntax and how they can be implemented in computer programming languages. Before we do so, let’s consider a hypothetical scenario to illustrate the power of lambda functions.
Imagine a software development project where multiple programmers are collaborating on writing code for an e-commerce website. Each programmer is responsible for developing specific functionalities, such as user authentication, product listing, and order processing. In this complex system, communication between different modules becomes crucial. Here is where lambda functions come into play.
Lambda functions offer several benefits in computer programming languages:
- Code conciseness: By eliminating the need to define full-fledged named functions, lambda functions allow developers to write shorter and more concise code snippets.
- Improved readability: With their compact syntax and ability to encapsulate functionality within a single expression, lambda functions enhance code readability by making it easier to understand the logic at hand.
- Flexibility in function passing: Lambda functions enable programmers to pass behavior or functionality as arguments to other functions effortlessly. This flexibility allows for greater modularity and reusability within the codebase.
- Efficient memory usage: Unlike traditional named functions that are stored permanently in memory once defined, lambda functions exist only when required and are garbage-collected automatically after execution. This efficient use of memory resources contributes to overall system performance.
Benefit | Description |
---|---|
Code Conciseness | Shorter and more concise code snippets |
Improved Readability | Easier understanding of logic |
Flexibility in Function Passing | Effortless passing of behavior/functionality |
Efficient Memory Usage | Optimal utilization of memory resources |
In summary, lambda functions provide numerous advantages in computer programming languages through their concise syntax, improved readability, flexible function passing capabilities, and efficient memory usage. Understanding these benefits will help programmers leverage the power of lambda functions in their code. In the following section, we will explore various use cases where lambda functions can be applied effectively to solve real-world problems and enhance software development processes.
Transitioning into the next section about “Use Cases of Lambda Functions,” let us now turn our attention to practical applications of these powerful tools.
Use Cases of Lambda Functions
Lambda Functions: The Power of Functions in Computer Programming Languages
Syntax of Lambda Functions (continued):
In the previous section, we discussed the syntax and structure of lambda functions. Now, let us explore some practical use cases that demonstrate the power and versatility of these functional constructs.
Imagine a scenario where you are developing a web application that requires sorting a list of user names alphabetically based on their last name. With traditional functions, you would need to define a separate function explicitly for this purpose. However, with lambda functions, you can achieve this task more concisely by creating an anonymous function inline.
To illustrate further, consider the following example:
user_names = ["John Doe", "Jane Smith", "Adam Johnson"]
sorted_names = sorted(user_names, key=lambda x: x.split(" ")[-1])
print(sorted_names)
This code snippet uses the sorted()
function along with a lambda function as its key
parameter. The lambda function splits each element in the user_names
list into individual words using the space character as a delimiter (x.split(" ")
). It then selects the last word ([-1]
) which represents the last name for each user. By passing this lambda function as an argument to sorted()
, we achieve our desired result – a sorted list of user names based on their last names.
The benefits of using lambda functions extend beyond just concise syntax and ease-of-use. Here are some advantages worth considering:
- Improved Readability: Lambda functions allow developers to write shorter and more focused code snippets within expressions or higher-order functions.
- Increased Productivity: Since they eliminate the need for defining named functions separately, lambda functions streamline development efforts by reducing unnecessary overhead.
- Enhanced Flexibility: Lambda functions provide flexibility when working with higher-order functions such as map(), filter(), or reduce(). They enable programmers to express complex operations succinctly.
Advantages of Lambda Functions |
---|
Improved Readability |
Increased Productivity |
Enhanced Flexibility |
In conclusion, lambda functions offer a concise and powerful way to define anonymous functions in computer programming languages. Their ability to simplify complex tasks and improve code readability makes them invaluable tools for developers.
Differences between Lambda Functions and Regular Functions
Example Scenario:
Imagine you are developing a web application that requires real-time data processing and analysis. One of the core functionalities of your application is to filter, transform, and aggregate large sets of data received from various sources. In this scenario, lambda functions can be highly beneficial.
Signpost 1 – Flexibility and Scalability:
Lambda functions offer developers the flexibility to write small, self-contained pieces of code that can be executed on demand without the need for defining separate functions or classes. This makes them particularly useful when dealing with ad-hoc operations or situations where creating dedicated functions would be impractical. Moreover, lambda functions provide scalability advantages as they can easily handle sudden spikes in workload by automatically scaling resources based on demand.
- Increased development efficiency
- Simplified code maintenance
- Enhanced productivity
- Improved resource utilization
Signpost 2 – Practical Applications:
The versatility of lambda functions enables their use across diverse domains and scenarios. For instance:
- Real-time event-driven architectures: Lambda functions can be employed to process incoming events or streams continuously.
- Serverless computing: By utilizing cloud platforms like AWS Lambda or Azure Functions, developers can focus solely on writing business logic while leaving infrastructure management to the platform provider.
- Data transformation and ETL (Extract, Transform, Load): Lambda functions enable seamless handling of complex data transformations before loading it into target systems.
Emotional Table:
Benefits | Description | Example |
---|---|---|
Reduced operational costs | Lambda functions help minimize expenses associated with server provisioning and maintenance. | Decreased monthly hosting fees |
Faster time-to-market | The ease of deploying lambda functions accelerates software deployment cycles. | New features released ahead of schedule |
Improved system reliability | Fault-tolerant nature ensures high availability even during peak usage periods. | Zero downtime during critical periods |
Enhanced user experience | Lambda functions allow for efficient processing, leading to faster response times and smoother user interactions. | Reduced waiting time for data retrieval |
Signpost 3 – Harnessing the Power:
By harnessing the power of lambda functions in computer programming languages, developers can unlock a wide range of possibilities. Whether it is real-time event processing or optimizing resource utilization, lambda functions offer an elegant solution that combines flexibility with scalability. In the following section, we will explore how different programming languages support lambda functions and showcase their unique features.
With a solid understanding of lambda function benefits and practical applications, let us now delve into the realm of implementing these powerful constructs across various programming languages.
Lambda Functions in Different Programming Languages
Having explored the concept of lambda functions and their applications in computer programming languages, it is now important to understand how they differ from regular functions. To illustrate this distinction, let us consider a hypothetical scenario where we are tasked with calculating the total price of items in a shopping cart.
One key difference between lambda functions and regular functions lies in their syntax. While regular functions have a defined name followed by parentheses for parameters, lambda functions do not require a name but instead consist of an anonymous function that can be assigned to a variable or passed as an argument to another function. In our case study, we may define a regular function named ‘calculateTotalPrice’ with parameters such as ‘cartItems’, which would perform the necessary calculations. On the other hand, a lambda function could be used within the code directly without having to define a separate function.
Another contrasting aspect is related to scope. Regular functions have their own local scope, whereas lambda functions inherit the scope from their surrounding environment. This means that variables outside the lambda function can also be accessed within its body. In our example, if there was a discount percentage variable declared outside both types of functions, it could easily be utilized within either one.
Furthermore, unlike regular functions that typically consist of multiple lines of code, lambda functions tend to be concise and often fit on a single line. The brevity of lambda expressions makes them well-suited for scenarios like filtering data or performing simple calculations quickly. This characteristic allows programmers to write cleaner and more readable code when appropriate.
To summarize:
- Syntax: Lambda functions lack names and utilize anonymous expressions.
- Scope: Lambda functions inherit their surrounding environment’s scope.
- Conciseness: They are commonly shorter than regular functions due to their focused purpose.
By understanding these differences between lambda and regular functions, developers can choose the most suitable approach based on specific requirements and coding style preferences. This knowledge empowers programmers to leverage the full potential of lambda functions in their respective programming languages, optimizing efficiency and code readability.