Higher-order functions: The Power of Functions in Programming Languages
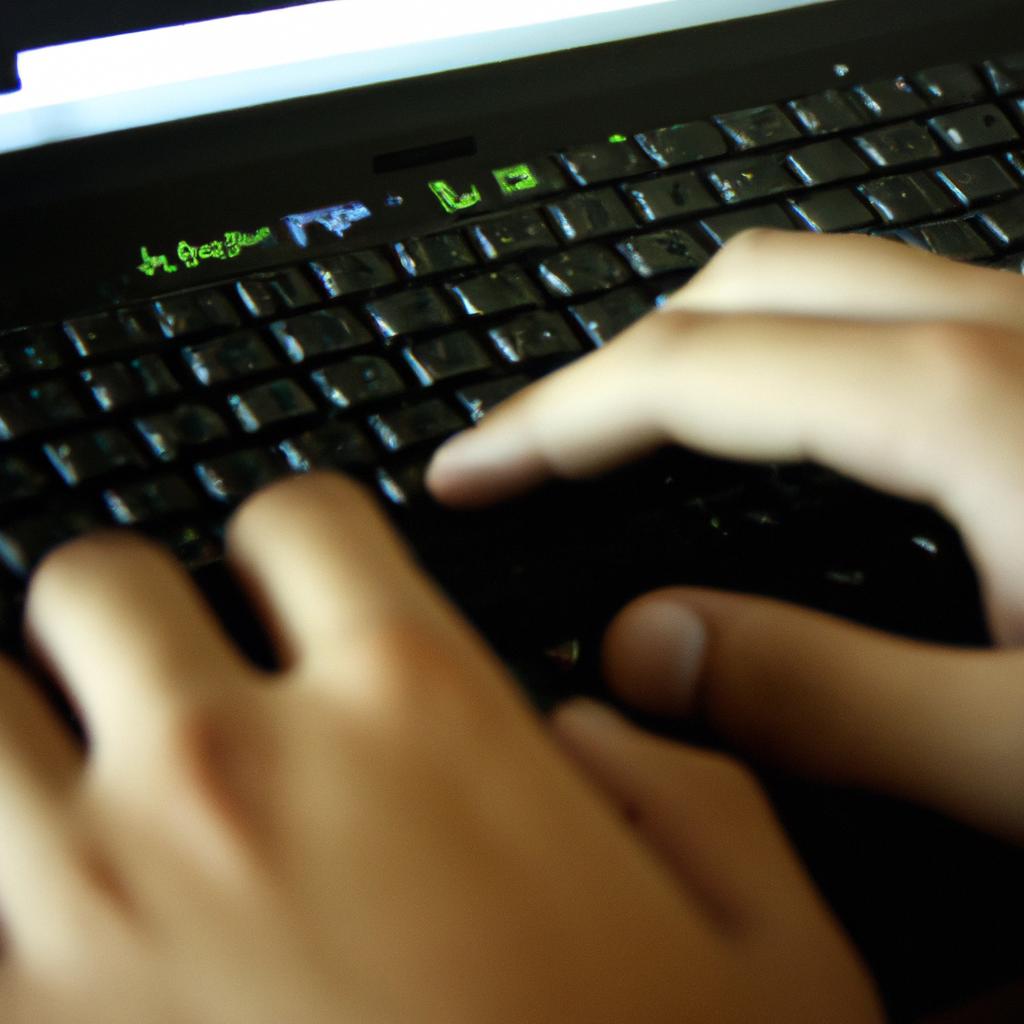
Higher-order functions are a fundamental concept in programming languages that allow for the manipulation and utilization of functions as first-class entities. These functions have the unique ability to accept other functions as arguments, return functions as results, or both. This powerful feature enables programmers to write more concise and flexible code by abstracting common patterns into reusable components.
To illustrate the significance of higher-order functions, consider a hypothetical scenario where a software developer is tasked with implementing a data processing system for an e-commerce platform. The system needs to perform various operations on customer data such as filtering out inactive users, calculating average purchase amounts, and generating personalized recommendations. By employing higher-order functions, the developer can create generic algorithms that take specific business logic — such as criteria for determining user activity status or recommendation generation rules — as input parameters. This modular approach not only simplifies development but also enhances maintainability and reusability of code across different parts of the application.
In this article, we will delve deeper into the power and versatility of higher-order functions in programming languages. We will explore their key characteristics, advantages, and use cases in real-world scenarios. Additionally, we will examine how popular programming languages like JavaScript, Python, and Scala embrace higher-order functions to enable developers to write elegant and efficient code. Lastly, we will provide practical examples and best practices for leveraging higher-order functions in your own projects.
Before diving into the details, let’s understand some fundamental concepts related to higher-order functions. In programming languages that support higher-order functions, functions are treated as first-class citizens, just like any other data type such as integers or strings. This means that functions can be assigned to variables, passed as arguments to other functions, and returned from functions.
One of the key advantages of higher-order functions is their ability to enable code reusability. By abstracting common patterns into higher-order functions, developers can write generic algorithms that can be applied to different contexts without having to rewrite the same logic multiple times. This not only reduces code duplication but also improves maintainability and readability by encapsulating functionality into small, self-contained units.
Higher-order functions also facilitate a more declarative style of programming. Instead of explicitly outlining step-by-step instructions for accomplishing a task, you can focus on describing what needs to be done without worrying about how it will be done. Higher-order functions allow you to pass in the necessary behavior as an argument, which makes code more expressive and easier to reason about.
The applications of higher-order functions are vast and span across various domains. Some common use cases include:
- Callbacks: In event-driven programming or asynchronous operations, callbacks are often used with higher-order functions to handle events or execute code once a certain operation is completed.
- Iteration: Higher-order functions like
map
,filter
, andreduce
enable efficient processing of collections by applying a given function to each element or filtering out specific elements based on a condition. - Function composition: Higher-order functions make it easy to combine multiple smaller functions into larger ones by chaining them together.
- Dependency injection: By passing dependencies as arguments through higher-order functions, you can achieve loose coupling between components and promote modularity and testability in your codebase.
- Memoization: Higher-order functions can be used to implement caching mechanisms that store the results of expensive computations for later reuse, improving performance.
JavaScript, Python, and Scala are popular programming languages that embrace higher-order functions. In JavaScript, functions are first-class objects, which makes it easy to pass them as arguments or return them from other functions. The Array.prototype
methods like map
, filter
, and reduce
are examples of higher-order functions widely used in JavaScript.
Python supports higher-order functions through its support for closures and lambda expressions. Functions like map
, filter
, and reduce
are also available in Python’s standard library.
Scala is a language that fully embraces functional programming concepts. It provides powerful tools for working with higher-order functions such as currying, partial application, and composition. Scala’s collections library offers a rich set of higher-order function combinators that enable expressive data manipulation.
In conclusion, higher-order functions play a crucial role in modern programming languages by providing flexibility, reusability, and expressiveness to developers. By understanding their principles and utilizing them effectively, you can write more elegant and efficient code while promoting modularity and maintainability in your projects.
Understanding higher-order functions
Imagine a scenario where you have been assigned the task of creating an application that calculates the total cost of a customer’s online shopping cart. As a programmer, you start by writing code to add up all the prices of the items in the cart and then apply any applicable discounts. However, as your project progresses, you realize that there are multiple ways to calculate discounts based on different criteria such as item types or customer loyalty levels. This is where higher-order functions come into play.
Higher-order functions, also known as functionals, are an essential concept in programming languages. They allow us to treat functions as first-class citizens – just like any other data type. In simpler terms, this means we can pass functions as arguments to other functions or return them as results from a function call.
To illustrate their significance, consider these practical applications:
- Modularity: Higher-order functions promote modular design by enabling developers to break down complex tasks into smaller, reusable building blocks. By passing different functions as arguments to a higher-order function, one can achieve flexibility and avoid repetitive code.
- Abstraction: With higher-order functions, programmers can define general-purpose algorithms that work with various input types simply by providing specific functions for processing those inputs.
- Code readability: Using higher-order functions often leads to more concise and expressive code compared to traditional imperative approaches. The functional style focuses on what needs to be done rather than how it should be done.
- Concurrency: Higher-order functions facilitate concurrent programming paradigms such as asynchronous event handling or parallel computation by allowing developers to create abstractions around operations performed on streams of events or data.
Table: Examples of Higher-Order Functions in Different Programming Languages
Language | Function Name | Description |
---|---|---|
JavaScript | map |
Transforms each element of an array using a provided mapping function and returns a new array. |
Python | filter |
Selects elements from an iterable based on a given predicate function and returns them as a list. |
Haskell | foldr |
Applies a binary operation to each element of a list, accumulating the results into a single value. |
Ruby | each_with_index |
Iterates over an enumerable object, passing both the current item and its index to a block. |
By understanding higher-order functions’ capabilities and their applications in various programming languages, developers can unlock powerful tools for code organization, abstraction, and scalability. In the subsequent section, we will explore the benefits of using higher-order functions.
Note: Higher-order functions are not limited to these specific examples or languages; they exist in numerous programming paradigms across different domains.
The concept of higher-order functions offers several advantages that enhance software development processes. From increased modularity to improved code readability, utilizing this functional programming feature has become crucial for modern-day programmers seeking efficient solutions to complex problems.
…
Benefits of using higher-order functions
Section H2: Exploring the Applications of Higher-Order Functions
Consider a scenario where you are developing a web application that involves processing large amounts of data. Traditionally, you would write separate functions to perform specific tasks such as filtering, mapping, and reducing this data. However, with higher-order functions, you can take your programming skills to the next level by working with functions as values in their own right.
One powerful example of using higher-order functions is when implementing a search feature on a website. By utilizing higher-order functions like filter
, map
, and reduce
, you can easily manipulate an array of objects representing various items for sale. For instance, imagine implementing a price filter that allows users to specify a maximum price they are willing to pay. With higher-order functions, it becomes possible to dynamically filter out all items exceeding the specified price range without writing repetitive code.
The benefits of incorporating higher-order functions into your programming repertoire are numerous:
- Increased code reusability: Higher-order functions allow you to abstract common patterns into reusable blocks of code, reducing redundancy.
- Enhanced expressiveness: Writing programs using higher-order functions often leads to more concise and expressive code due to their ability to encapsulate complex logic within simple function calls.
- Improved readability: By leveraging built-in higher-order functions or creating custom ones tailored to your needs, you can make your code more readable and easier for others (and even yourself) to understand.
- Flexibility and adaptability: Higher-order functions provide flexibility in how you structure your code by allowing behavior customization through the use of callback functions.
Benefit | Description |
---|---|
Code reusability | Avoid repetitive coding patterns by extracting them into reusable functions. |
Expressive syntax | Write cleaner and shorter code that conveys intent effectively. |
Readable and understandable | Improve legibility and comprehension for both developers and collaborators. |
Flexible customization | Adapt functions to different scenarios by passing in customizable behaviors. |
In conclusion, higher-order functions empower programmers with a powerful toolset that enhances code reusability, expressiveness, readability, and flexibility. By abstracting common patterns into reusable blocks of code, developers can create more efficient programs while simplifying their implementation.
[Examples of higher-order functions in programming]
Examples of higher-order functions in programming
Imagine a scenario where you are developing a web application that requires sorting and filtering large sets of data. Without higher-order functions, this task can become cumbersome and time-consuming. However, by harnessing the power of higher-order functions, you can significantly simplify your code and enhance its functionality.
One practical example of using higher-order functions is in implementing search functionalities on e-commerce websites. Consider an online marketplace with thousands of products. To allow users to easily find what they are looking for, it becomes essential to provide them with efficient search options. By utilizing higher-order functions such as filter
or find
, developers can create dynamic search filters that match specific criteria provided by the user. This capability not only improves the user experience but also streamlines the development process.
When exploring the applications of higher-order functions further, it is helpful to understand some key benefits they offer:
- Code reusability: Higher-order functions enable us to define generic operations that can be applied to various data sets or scenarios.
- Modularity: By breaking down complex tasks into smaller functions, we achieve modularity, allowing for easier debugging and maintenance.
- Flexibility: With higher-order functions, programmers have greater flexibility in designing their programs since these functions can be composed and combined effectively.
- Abstraction: Higher-order functions promote abstraction by separating control flow from implementation details, making code more concise and readable.
To illustrate how diverse their applications can be across different programming paradigms, let’s consider a table showcasing some common use cases for higher-order functions:
Paradigm | Use Case |
---|---|
Functional Programming | Mapping over collections |
Object-Oriented Programming | Implementing callback mechanisms |
Event-driven Programming | Handling asynchronous events |
Reactive Programming | Composing reactive streams |
Incorporating higher-order functions into your programming arsenal unlocks a realm of possibilities, allowing you to write more elegant and efficient code. However, it is essential to address common misconceptions about their usage in order to fully grasp their potential.
Transitioning seamlessly into the subsequent section on “Common misconceptions about higher-order functions,” let us now examine some of these misunderstandings and clarify them for better understanding.
Common misconceptions about higher-order functions
Transitioning seamlessly from the previous section’s exploration of examples, we can now delve into the numerous benefits that higher-order functions bring to programming languages. To illustrate these advantages, let us consider an example scenario where a software developer is tasked with implementing a sorting algorithm for a large dataset.
In this hypothetical case, our developer could choose to write a traditional sorting function that explicitly defines the comparison logic within it. However, by utilizing higher-order functions, they can take advantage of the flexibility and power they offer. Instead of hard-coding the comparison logic, our developer could pass a comparator function as an argument to their sorting function. This allows them to sort various types of data (e.g., numbers or strings) using different criteria without having to rewrite multiple versions of the same sorting algorithm.
The use of higher-order functions provides several key benefits in such scenarios:
- Code Reusability: By decoupling functionality through passing functions as arguments, developers can reuse existing code components across different parts of their application.
- Reduced Code Duplication: Higher-order functions allow developers to avoid duplicating similar code patterns by abstracting common operations into reusable functions.
- Increased Flexibility: With the ability to pass different functions as arguments, applications become more adaptable and can easily change behavior based on varying requirements.
- Enhanced Readability: Utilizing higher-order functions promotes cleaner and more concise code by separating concerns and making each piece easier to understand.
Indeed, embracing higher-order functions empowers developers to write efficient programs while promoting modularity and maintainability. These advantages make them invaluable tools when designing complex systems that require flexible yet scalable solutions.
Moving forward, let us explore some challenges and considerations that programmers should keep in mind when working with higher-order functions.
Challenges and considerations when using higher-order functions
Consider a scenario where you are building a web application that requires complex data manipulation and filtering. You have a dataset containing information about various products, such as their names, prices, and categories. To extract specific subsets of this data based on different criteria (e.g., price range, category), you could write separate functions for each criterion. However, with higher-order functions, you can create more flexible and reusable code by abstracting away common patterns.
One example of using higher-order functions in this context is implementing a filter function. This function takes an array of items and a predicate function as arguments and returns a new array containing only the items that satisfy the given condition defined by the predicate. By passing different predicates to the filter function, you can easily obtain subsets of the original dataset without duplicating logic or writing additional code.
Higher-order functions offer several benefits that enhance code modularity and maintainability:
- Code Reusability: With higher-order functions, developers can encapsulate commonly used patterns into reusable abstractions. These abstractions allow them to apply similar transformations across different datasets or even different parts of an application.
- Flexibility: The ability to pass functions as arguments enables dynamic behavior within programs. Higher-order functions empower developers to adapt their code at runtime based on changing requirements or user input.
-
Readability: By utilizing higher-order functions, developers can express program flow in a concise manner. Instead of dealing with low-level iteration constructs like
for
loops, they can utilize built-in higher-order functions provided by programming languages to perform operations such as mapping or reducing over collections. - Testability: Higher-order functions promote test-driven development practices by providing clear separation between core functionality and auxiliary concerns. Developers can focus on testing individual components in isolation before composing them together using higher-order functions.
Benefit | Description |
---|---|
Code Reusability | Encapsulate commonly used patterns into reusable abstractions to apply similar transformations across datasets. |
Flexibility | Adapt code at runtime based on changing requirements or user input, allowing dynamic behavior within programs. |
Readability | Express program flow concisely using higher-order functions instead of low-level iteration constructs like for loops. |
Testability | Promote test-driven development by separating core functionality from auxiliary concerns for easier component testing. |
Exploring advanced techniques with higher-order functions
Transitioning from the challenges faced while employing higher-order functions, we now delve into exploring advanced techniques that leverage the full potential of these versatile constructs. To illustrate their efficacy, let us consider a hypothetical scenario involving a web application that requires dynamic user interface updates based on real-time data.
In this case study, imagine a financial analytics platform where users can monitor stock market trends. By utilizing higher-order functions, developers can implement a feature that enables the display of live charts showcasing multiple stocks simultaneously. This would involve creating a function that accepts another function as an argument to fetch real-time data for each stock selected by the user. Subsequently, this fetched data is processed and rendered onto the user interface dynamically.
To further highlight the benefits of leveraging higher-order functions, we present below a bullet point list summarizing key advantages:
- Enhanced code reusability and modularity
- Increased flexibility to adapt to changing requirements
- Facilitated implementation of complex algorithms or workflows
- Improved readability and maintainability through abstraction layers
Moreover, it is worth noting that different programming languages provide varying degrees of support for higher-order functions. To aid in understanding how popular languages embrace this concept, we present a table highlighting notable examples:
Language | Support for Higher-Order Functions |
---|---|
JavaScript | Native support; extensively used |
Python | First-class citizens; widely adopted |
Haskell | Core language feature; functional paradigm |
Ruby | Strong support via blocks and lambdas |
By embracing these advanced techniques made possible by higher-order functions, developers gain powerful tools to solve intricate problems more efficiently. They can architect elegant solutions by composing smaller reusable units rather than dealing with monolithic codebases. This fosters a more agile development process and empowers programmers to create robust software systems capable of adapting to evolving user requirements.
In summary, the exploration of advanced techniques with higher-order functions unlocks new possibilities in programming languages. By employing these constructs effectively, developers can enhance code reusability, adaptability, and overall maintainability. The following section will delve deeper into specific methodologies that further extend the capabilities of higher-order functions within various contexts.