Control Flow in Computer Programming Languages: And Utilizing the Fundamental Concepts
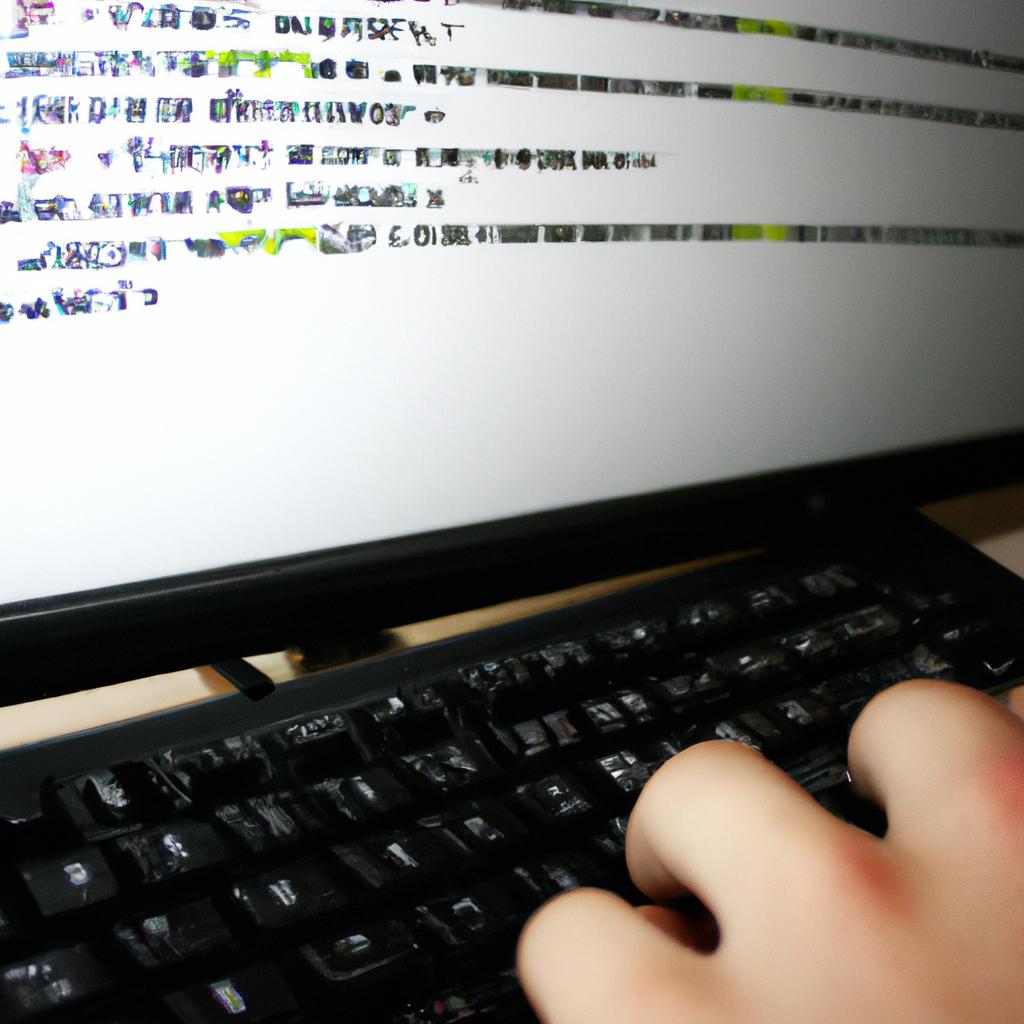
Control flow is a fundamental concept in computer programming languages that governs the order and execution of statements within a program. It determines how instructions are carried out, allowing programmers to control the logical flow of their code. By understanding and effectively utilizing control flow mechanisms, developers can create efficient and reliable software applications.
Consider the scenario of an e-commerce website where customers make online purchases. In this case, control flow plays a crucial role in managing various aspects of the purchasing process. For instance, upon receiving an order, the program needs to verify if the customer’s payment information is valid before proceeding with shipping confirmation. This validation step requires conditional statements within the control flow structure to check for potential errors or discrepancies in real-time.
In this article, we will delve into the intricacies of control flow in computer programming languages and explore its fundamental concepts. We will examine different control structures such as loops, conditionals, and function calls, discussing how each contributes to controlling program execution. Furthermore, we will investigate common pitfalls and best practices when working with control flow to ensure optimal code performance and maintainability.
Logical Constructs in Programming
One of the fundamental concepts in computer programming is the use of logical constructs to control the flow of execution. These constructs, such as if statements and loops, allow programmers to make decisions based on certain conditions or repeat a set of instructions multiple times. To better understand the importance and utilization of these logical constructs, let us consider an example scenario.
Imagine a program that manages student grades. In this program, there is a need to determine whether a student has passed or failed a course based on their final grade. By using an if statement, the program can evaluate the condition “if (finalGrade >= 60)”, where “finalGrade” represents the numerical value obtained by the student. If this condition evaluates to true, then it means that the student has passed; otherwise, they have failed. This simple yet powerful construct allows for dynamic decision-making within programs.
To further illustrate the significance of logical constructs in programming, here are some emotional responses often evoked when working with them:
- Relief: Logical constructs provide relief by allowing programmers to automate decision-making processes and reduce manual intervention.
- Confidence: The ability to write code with logic instills confidence in programmers as they know their programs will respond accordingly.
- Efficiency: Utilizing logical constructs improves efficiency by eliminating repetitive tasks through loops and conditional statements.
- Organization: By incorporating logical constructs into their code, programmers can create structured and organized solutions.
In addition to understanding these emotional responses, it is helpful to visualize how logical constructs work together. Consider the following table showcasing different scenarios based on two variables: age and gender.
Age | Gender | Eligible for Voting? |
---|---|---|
18 | Male | Yes |
16 | Female | No |
22 | Male | Yes |
14 | Female | No |
This table demonstrates how logical constructs can be used to make decisions based on specific conditions. By applying if statements or other decision-making constructs, a program can determine the eligibility of individuals for voting in this case.
In summary, logical constructs play a vital role in computer programming by allowing programmers to control the flow of execution and make decisions based on various conditions. Through the use of if statements and loops, programmers can create dynamic solutions that automate processes, instill confidence, improve efficiency, and promote organization within their code. With an understanding of these concepts, we can now delve into exploring conditional execution in code.
Conditional Execution in Code
Transitioning from the previous section on logical constructs, we now delve into another crucial aspect of computer programming: control flow structures. These structures dictate how instructions are executed within a program and enable programmers to create dynamic and flexible code. To illustrate their importance, let’s consider an example scenario where an e-commerce website needs to determine whether a user qualifies for a discount based on their purchase history.
One common type of control flow structure is conditional execution, which allows specific blocks of code to be executed only if certain conditions are met. In our example, the website would use conditional statements to check if the user has made enough purchases to qualify for a discount. If the condition evaluates to true, the appropriate block of code will execute; otherwise, it will be skipped entirely. This enables programs to make decisions and adapt their behavior based on different input or data states.
Another essential control flow structure is iteration or looping, allowing repetitive execution of a block of code until a particular condition is no longer satisfied. For instance, imagine that our hypothetical e-commerce website wants to display all purchased items by repeatedly fetching them from a database until there are no more records remaining. Loops provide an efficient way to automate such tasks without writing redundant code.
Control flow structures offer several benefits in programming:
- Enhance readability: By organizing code logically using conditionals and loops, developers can easily understand complex algorithms.
- Increase efficiency: Conditional execution allows programs to perform selective actions while skipping unnecessary steps, optimizing performance.
- Enable interactivity: Iteration provides opportunities for users’ input or external events to influence program behavior dynamically.
- Facilitate error handling: By incorporating decision-making mechanisms through control flow structures, programmers can handle exceptional cases gracefully.
To further emphasize these advantages visually:
Benefit | Description |
---|---|
Readability | Organized code improves understanding |
Efficiency | Selective execution optimizes performance |
Interactivity | Dynamic behavior based on user input or external events |
Error Handling | Graceful handling of exceptional cases |
In conclusion, control flow structures are fundamental concepts in programming languages that enable developers to create versatile and adaptive code. By employing conditional statements and loops, programmers can make decisions, automate repetitive tasks, enhance readability, increase efficiency, promote interactivity, and handle errors effectively. Next, we will explore the concept of iterating with loops and its significance within computer programming.
Moving forward into our discussion about “Iterating with Loops,” let’s explore how this construct allows for repeated execution of specific code blocks.
Iterating with Loops
Transition from Previous Section:
Having explored conditional execution in code, we now turn our attention to another vital aspect of programming – iterating with loops. Loops serve as powerful tools for repeating a set of instructions multiple times, enabling developers to automate repetitive tasks and efficiently process large amounts of data. Understanding how control flow works within loops is essential for writing efficient and effective programs.
The Power of Loops
To illustrate the significance of loops, consider the following scenario: imagine you are building a program that needs to calculate the sum of all numbers between 1 and 1000. Without using any form of iteration, one would have to write out each number individually and then manually perform the addition operation. However, by utilizing a loop construct such as a ‘for’ or ‘while’ loop, this task can be accomplished rapidly and accurately with just a few lines of code.
Loops allow programmers to execute a block of code repeatedly until certain conditions are met or until they explicitly break out of the loop. They provide flexibility in controlling the flow of statements based on logical expressions or pre-defined iterations. Here are some key benefits associated with leveraging loops:
- Efficiency: By automating repetitive tasks, loops help reduce redundancy in code.
- Productivity: Developers can accomplish more in less time by applying looping constructs.
- Scalability: With loops, it becomes easier to handle larger datasets or carry out complex operations.
- Maintainability: Using loops results in concise and readable code that is easier to understand and modify.
Benefits of Looping |
---|
Efficient execution |
Increased productivity |
Scalable solution |
Improved code maintainability |
Types of Loops
In most programming languages, there are two commonly used types of loops: ‘for’ loops and ‘while’ loops. Each type serves its purpose in different scenarios and provides distinct approaches to iterating through code.
Type of Loop | Description |
---|---|
For Loop | A ‘for’ loop repeatedly executes a block of code for a fixed number of times. It uses an index variable to control the iteration, incrementing or decrementing it after each execution until a specified condition is met. |
While Loop | A ‘while’ loop continues executing a block of code as long as a given condition remains true. The loop checks the condition at the beginning of each iteration, allowing flexibility in handling unknown or changing conditions. |
By understanding how these loops operate and when to use them appropriately, programmers can harness their power to create efficient and dynamic programs.
Transition to Next Section:
As we have explored the concept of control flow within loops, it is important to note that unexpected situations may arise during program execution. In our next section on “Handling Exceptions in Programs,” we will delve into techniques for gracefully managing errors and exceptions that occur while running code. By understanding how to handle such situations effectively, developers can ensure their programs are robust and reliable.
Handling Exceptions in Programs
Section H2: Iterating with Loops
In the previous section, we explored the concept of iterating with loops in computer programming languages. We saw how loops allow us to repeat a block of code multiple times, thereby enabling efficient and concise coding practices. Now, let’s delve into another crucial aspect of programming – handling exceptions.
Imagine you are developing an application that processes user data from various sources. One day, while running your program, it encounters a situation where the input file is missing or corrupted. This unexpected event can cause your program to crash if not handled properly. Here comes the significance of Exception handling techniques within control flow structures.
Exception handling involves identifying and responding to exceptional conditions or errors that may occur during program execution. By incorporating error-handling mechanisms like try-catch blocks, you can gracefully handle such situations instead of abruptly terminating the program. Exceptional events could range from invalid user inputs to network failures or system resource unavailability.
- Avoid program crashes and enhance stability.
- Improve user experience by providing meaningful error messages.
- Enable graceful recovery from unforeseen circumstances.
- Maintain code readability and modularity through encapsulation.
Additionally, let’s illustrate some commonly encountered exceptions using a three-column and four-row table:
Exception | Description | Common Causes |
---|---|---|
NullPointerException | Trying to access a null object reference | Forgetting to initialize an object |
ArrayIndexOutOfBoundsException | Accessing an array index outside its bounds | Misusing loop indices |
FileNotFoundException | Attempting to open a non-existent file | Providing incorrect file path |
ArithmeticException | Performing illegal arithmetic operations | Division by zero |
As we conclude our discussion on exception handling within control flow structures, let’s now shift our focus to understanding control flow itself. Control flow refers to the order in which statements are executed within a program. By comprehending control flow mechanisms and techniques, programmers can effectively guide their programs’ execution paths based on certain conditions or events.
In this next section, we will explore various control flow constructs such as conditional statements (if-else), switch-case statements, and function calls. Understanding these fundamental concepts will empower you to design robust algorithms and write efficient code that meets specific requirements while maintaining program integrity. So, let’s embark on an exciting journey into the realm of control flow!
Understanding Control Flow
Transitioning from the previous section on handling exceptions, let us now delve into a crucial aspect of computer programming languages – control flow. Control flow refers to the order in which statements are executed within a program. By understanding and effectively utilizing control flow mechanisms, programmers can guide the execution of their code based on specific conditions or events.
To illustrate this concept, consider a hypothetical scenario where we have developed a software application for an online bookstore. When a user searches for books by entering keywords, our program needs to display only those books that match the search criteria. To achieve this, we would utilize control flow statements such as if-else and switch-case to filter out irrelevant book entries from being displayed.
Control flow statements offer several advantages when applied correctly:
- They allow programs to make decisions based on certain conditions.
- They enable looping constructs that repeat blocks of code until specific conditions are met.
- They facilitate branching, wherein different actions can be taken depending on various scenarios.
- They provide error-handling capabilities through exception handling mechanisms.
Let’s examine these benefits further using a table:
Benefit | Description |
---|---|
Decision-making | Control flow statements help programs decide between multiple paths of execution. |
Looping constructs | These structures allow repetition of tasks until predetermined conditions are satisfied. |
Branching | Programs can take different routes based on varying circumstances or inputs. |
Error handling | Exception handling allows programs to handle errors gracefully instead of crashing unexpectedly. |
As we conclude this section exploring control flow concepts, it is evident that mastering control flow is essential for effective program design and execution. The upcoming section will focus specifically on one such statement – if statements. By studying how if statements work and their various applications, you will gain valuable insights into controlling the behavior of your programs based on specific conditions.
Transitioning to the subsequent section, let us now delve into exploring if statements and their significance in computer programming languages.
Exploring if Statements
Case Study: Imagine a scenario where you are tasked with analyzing sales data for a large retail company. The dataset contains information about each transaction, including the date, product sold, and quantity. Your goal is to calculate the total revenue generated by each product category over a specified time period. This task requires processing thousands of records efficiently and accurately.
To achieve this, we can utilize loops as an indispensable tool in control flow within computer programming languages. A loop allows us to repeat a set of instructions multiple times until a certain condition is met or for a specific number of iterations. By employing loops effectively, we can automate repetitive tasks and handle large amounts of data swiftly and systematically.
When working with loops in control flow, it is essential to understand their various types and how they differ in functionality:
- For Loop: Executes a block of code repeatedly based on a predetermined increment or decrement.
- While Loop: Repeats a block of code while a given condition remains true.
- Do-While Loop: Similar to the while loop but guarantees at least one execution before checking the condition again.
The following table highlights some key characteristics and use cases for these different types of loops:
Loop Type | Key Characteristics | Common Use Cases |
---|---|---|
For Loop | Allows iteration over a range or sequence of values | Summing elements in an array |
While Loop | Continues looping as long as a certain condition holds | Reading input until reaching end-of-file |
Do-While | Always executes at least once regardless of condition | Validating user input |
By grasping the concept behind each type of loop and understanding when to apply them appropriately, programmers gain immense power to manipulate data efficiently and solve complex problems methodically.
Transition into subsequent section – “The Power of for Loops”: Moving on from exploring the different types of loops, we will now delve into the specific capabilities and advantages offered by for loops in control flow.
The Power of for Loops
In the previous section, we delved into the concept of if statements in computer programming languages. Now, let us further expand our understanding by exploring their practical application and impact on control flow.
To illustrate this, consider a scenario where an e-commerce website offers discounts based on the total purchase amount. If the total exceeds $100, a 10% discount is applied; otherwise, no discount is given. By using an if statement, the program can evaluate whether the condition of the total exceeding $100 is true or false and execute the appropriate code block accordingly.
Utilizing if statements provides several advantages:
-
Decision making: If statements allow for conditional execution of different sections of code based on specified conditions. This enables programmers to create dynamic programs that adapt to various scenarios.
-
Code optimization: By employing if statements effectively, unnecessary computations can be avoided. For instance, in our e-commerce example, there would be no need to calculate a discount if the total does not exceed $100.
-
Error handling: If statements are instrumental in handling errors and exceptions gracefully. They enable programmers to anticipate potential issues and implement customized error-handling mechanisms when specific conditions occur.
-
User interaction: With if statements, programs can interact with users more intuitively by responding differently based on user input or system states. This enhances user experience and makes applications more engaging.
Condition | Action |
---|---|
True | Execute corresponding code block |
False | Skip executing corresponding code block |
In summary, If Statements serve as crucial building blocks for controlling program flow in computer programming languages. Their versatility allows programmers to make decisions, optimize code execution, handle errors efficiently, and enhance user interactions.
Next Section – The Power of for Loops
Utilizing the while Loop
Now, let’s delve into the concept of utilizing the while loop in computer programming. To illustrate its significance and practical application, consider a hypothetical scenario where you are developing a program to simulate a traffic light system. In this case, the while loop proves invaluable as it allows for continuous monitoring and control of the traffic light sequence until certain conditions are met.
One advantage of using a while loop is its flexibility in handling situations where the number of iterations cannot be predetermined. This makes it particularly useful when dealing with real-time data or unpredictable events. The ability to iterate indefinitely until specific conditions are satisfied ensures that your program can adapt dynamically to changing circumstances.
To further highlight the versatility of the while loop, here are some key points to consider:
- Efficient resource management: By incorporating conditional statements within the loop, you can optimize resource allocation and prevent unnecessary processing.
- Continuous monitoring: The while loop provides an effective means of continuously checking for updates or changes in variables or data sets.
- Iterative problem-solving: Through repeated execution of code blocks, complex problems can be broken down into simpler steps, making them more manageable.
- Event-driven programming: The while loop enables event-driven programming paradigms by allowing programs to respond promptly to external stimuli or user inputs.
Advantages | Limitations | Use Cases |
---|---|---|
Provides flexibility | Prone to infinite loops if not carefully managed | Real-time systems |
Efficient resource management | Requires careful consideration to ensure termination | Event-driven programming |
Allows continuous monitoring and tracking | Can lead to repetitive code if not properly structured | Iteratively solving complex problems |
Enables iterative problem-solving | May have performance implications depending on implementation | Situations requiring dynamic adaptation based on changing input |
In summary, harnessing the potential of the while loop in computer programming empowers developers to build programs that can adapt and respond dynamically to changing conditions. Its flexibility, continuous monitoring capabilities, and iterative problem-solving approach make it an invaluable tool for a wide range of applications.
Switch Statements: A Versatile Tool
Utilizing the while Loop Effectively
In order to maximize the potential of control flow in computer programming, it is important to explore and understand various tools available. One such tool that can greatly enhance program execution is the while loop. The while loop allows for repetitive execution of a block of code as long as a specified condition remains true.
To illustrate this concept, let’s consider a hypothetical scenario where we have an online marketplace with multiple sellers who need their products listed on the platform. By utilizing a while loop, we can automate the process of listing these products until all sellers’ inventories are uploaded. This not only saves time but also ensures accuracy and consistency throughout the product listings.
When using the while loop effectively, there are several key considerations to keep in mind:
- Establishing appropriate termination conditions or exit strategies to prevent infinite loops.
- Ensuring proper initialization and updating of variables within the loop body.
- Handling exceptions or errors that may arise during execution.
- Optimizing performance by minimizing unnecessary iterations.
These considerations highlight the significance of understanding how to utilize control structures like the while loop efficiently. By adhering to best practices in coding, programmers can improve both productivity and overall program reliability.
Now that we have explored the utilization of while loops, another powerful construct worth examining is switch statements. Switch statements allow for more structured decision-making based on different cases or values provided as input. They offer an alternative approach to nested if-else statements and can lead to cleaner and more readable code.
A common use case for switch statements is handling menu options in software applications. For instance, imagine a restaurant ordering system with a variety of dishes categorized into different types (e.g., appetizers, main courses, desserts). Using switch statements enables developers to map user inputs to specific actions associated with each type of dish quickly and efficiently.
By employing Switch Statements judiciously, programmers gain greater flexibility and control over the flow of their programs. This construct helps improve code readability, reduces complexity, and enhances maintainability by providing a concise way to handle multiple alternative paths.
Transitioning into error handling techniques, it is important to address potential issues that may arise during program execution. One widely-used approach for managing errors in many programming languages is the try-catch block. This mechanism allows developers to anticipate and handle exceptions gracefully, ensuring that programs continue running smoothly even when unexpected errors occur.
Understanding proper error handling techniques not only prevents crashes or abrupt terminations but also provides opportunities for graceful recovery or appropriate cleanup actions before exiting the program altogether.
Error Handling with try-catch
Error handling is a crucial aspect of programming that ensures the smooth execution and reliability of software. By anticipating and dealing with potential errors, developers can prevent unexpected program crashes or undesired behavior. One example illustrating the significance of error handling involves an e-commerce website that processes online orders. Without proper error handling, if there was an issue with processing payment for a customer’s order, such as a credit card decline, the system may fail to handle this error gracefully, causing frustration for both customers and administrators.
To effectively handle errors in programming languages, try-catch blocks are commonly employed. These constructs allow developers to catch exceptions thrown during runtime and perform appropriate actions based on the type of exception encountered. Here are some key reasons why error handling using try-catch blocks is essential:
- Ensures graceful degradation: If an exceptional situation arises during program execution, it is crucial to handle it properly instead of allowing the entire application to crash abruptly.
- Enhances debugging: Through effective error handling techniques like printing stack traces or logging relevant information about caught exceptions, developers gain valuable insight into what went wrong within their code.
- Enables recovery: In situations where recovering from an exception is possible (e.g., reconnecting to a database after a temporary network failure), error handling provides opportunities for corrective measures.
- Improves user experience: Properly handled errors present meaningful messages or alternative paths for users when something unexpected occurs, enhancing their overall satisfaction with the software.
Exception Type | Description | Example |
---|---|---|
ArithmeticException | Occurs when mathematical operations encounter invalid input | Dividing by zero |
NullPointerException | Indicates that an object reference points to null | Accessing a null object’s method |
IOException | Represents input/output-related issues | File not found |
IllegalArgumentException | Indicates that a method has been passed an illegal or inappropriate argument | Passing negative values to a method expecting positive numbers |
In summary, error handling is a critical aspect of programming that ensures the reliability and user-friendliness of software. By using try-catch blocks, developers can gracefully handle exceptions, enhancing debugging capabilities and allowing for potential recovery from exceptional situations. Properly implementing error handling techniques not only prevents crashes but also improves the overall user experience by providing meaningful feedback when errors occur.
Transitioning into the subsequent section about “Visualizing Control Flow Graphs,” we can explore how visual representations aid in understanding program execution paths and further enhance our comprehension of control flow in computer programs.
Visualizing Control Flow Graphs
Building upon the understanding of error handling with try-catch, we now delve into another crucial aspect of control flow in computer programming languages. In this section, we explore the significance and visual representation of control flow graphs.
Control Flow Graphs:
To illustrate the concept of control flow graphs, let us consider a hypothetical scenario where a programmer is developing an application to simulate traffic signals at an intersection. The program needs to determine which lights should be active based on various conditions such as time of day, traffic volume, and emergency situations. By analyzing the code for this application, we can construct a control flow graph that visually represents how the execution flows from one statement to another.
A control flow graph captures all possible paths that a program can take during its execution. It consists of nodes representing individual statements or blocks of code, connected by edges denoting the order in which these statements are executed. This graphical representation allows programmers to analyze complex programs more easily and identify potential issues like infinite loops, unreachable code, and unexpected behavior.
The benefits of utilizing control flow graphs in programming are numerous:
- Improved code comprehension: Control flow graphs provide a visual overview of program logic, helping developers understand how different parts interact and aiding in troubleshooting.
- Enhanced debugging capabilities: By examining the control flow graph during debugging sessions, programmers can trace through their code step-by-step and pinpoint areas causing errors or undesired outcomes.
- Increased maintainability: Visualizing control flow enables easier identification of redundant or duplicated code segments, fostering cleaner and more efficient software development practices.
- Facilitated collaboration: Sharing control flow graphs with team members promotes effective communication about program structure and functionality.
Benefit | Emotional Response |
---|---|
Improved Code Comprehension | Clarity |
Enhanced Debugging Capabilities | Confidence |
Increased Maintainability | Efficiency |
Facilitated Collaboration | Teamwork |
In the subsequent section, we will explore how to apply these fundamental concepts of control flow in programming through practical examples and exercises. Understanding the control flow graph is a vital step towards becoming proficient in writing reliable and efficient code.
Moving forward, let us now examine how to apply these fundamental concepts of control flow in programming when developing real-world applications.
Applying Fundamental Concepts in Programming
Section H2: Applying Fundamental Concepts in Programming
Transitioning from the previous section on visualizing control flow graphs, we now delve into the practical implementation of fundamental concepts in programming. To illustrate this, let’s consider a hypothetical scenario where we are developing a simulation game that involves navigating a maze. In order to accomplish this task successfully, various programming techniques and concepts need to be applied.
One crucial aspect when working with control flow in programming is error handling. When designing our maze navigation program, it is essential to anticipate and handle potential errors or exceptions that may occur during runtime. By using mechanisms such as try-catch blocks or conditionals, we can ensure graceful handling of unexpected situations, ensuring smooth execution of our code.
Another important concept is data structures. A well-designed maze navigation program would utilize appropriate data structures like stacks or queues to keep track of visited locations or store information about possible paths. These data structures enable efficient retrieval and manipulation of data, improving overall performance and accuracy within the simulation.
Furthermore, modularization plays a significant role in creating maintainable and reusable code. By breaking down complex tasks into smaller functions or modules, we enhance readability and promote code reusability. For instance, dividing the maze navigation program into separate functions for movement validation, path finding algorithms, and graphical representation allows us to isolate different concerns and simplify debugging processes.
- Increased code reliability through thorough error handling
- Enhanced efficiency by utilizing appropriate data structures
- Improved maintainability through modularization
- Simplified debugging process with isolated concerns
Additionally, presenting information visually can further engage readers. Here is a 3-column x 4-row table demonstrating how each concept contributes positively to the development process:
Concept | Benefit | Example |
---|---|---|
Error Handling | Ensures smooth execution of code | Gracefully recovering from unexpected errors |
Data Structures | Improves performance and accuracy | Efficiently storing visited locations or paths |
Modularization | Enhances maintainability and reusability | Isolating movement validation function |
In summary, applying fundamental concepts in programming is crucial to developing robust and efficient software solutions. By incorporating error handling mechanisms, utilizing suitable data structures, and implementing modularization techniques, we can create well-structured programs that are easier to debug, maintain, and reuse.