Variables: The Crucial Building Blocks of Computer Programming Languages
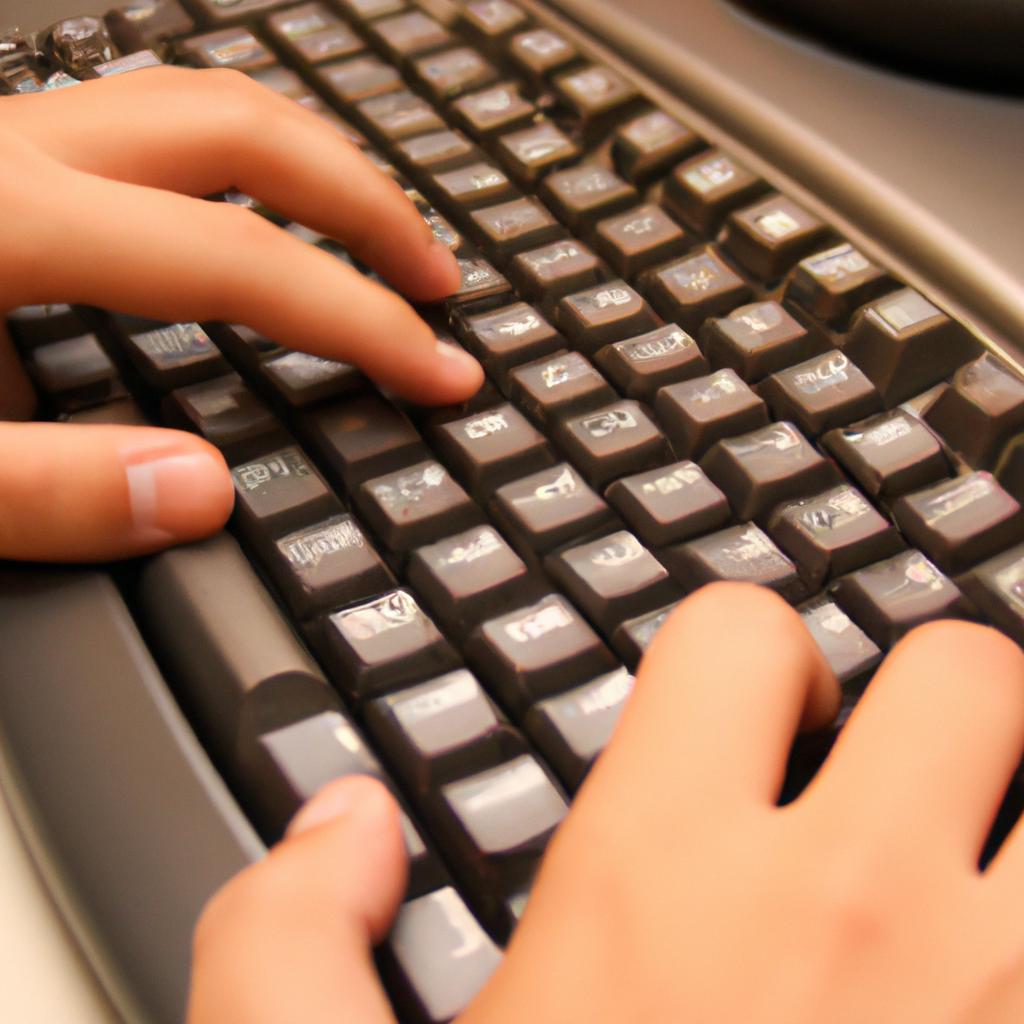
Variables are the fundamental components of computer programming languages, playing a crucial role in storing and manipulating data. Like containers that hold different types of information, variables enable programmers to create dynamic and interactive applications. For instance, consider a hypothetical scenario where a software developer is tasked with creating a weather application. Through the use of variables, they can store and update data such as temperature, humidity levels, and wind speed in real-time.
In the realm of computer programming languages, variables serve as placeholders for values that may change over time or during program execution. By assigning names to these placeholders, developers can easily refer to them throughout their code, facilitating efficient storage and retrieval of information. Additionally, variables allow programmers to perform various operations on the stored data by applying mathematical calculations or logical comparisons. This flexibility enables the creation of programs that respond dynamically to user inputs or changing environmental conditions. Consequently, understanding how variables work is paramount for aspiring programmers aiming to build robust and adaptable software solutions.
Understanding the importance of variables in programming
To grasp the significance of variables in computer programming, it is essential to comprehend their fundamental role as building blocks within programming languages. By allowing programmers to store and manipulate data values, variables serve as placeholders that enable dynamic execution and efficient problem-solving.
Consider a hypothetical scenario where a programmer endeavors to create a simple calculator program. Without utilizing variables, the task becomes arduous and impractical. Instead, by incorporating variables like “num1” and “num2,” one can easily store user input for numerical operands, perform operations on these values using mathematical operators such as addition or subtraction, and display the results promptly. This example underscores how variables facilitate streamlined calculation processes while enhancing code readability.
Understanding the importance of variables goes beyond mere convenience; they also play a pivotal role in optimizing program functionality through their flexibility and adaptability. Here are several key reasons why variables hold such paramount value:
- Efficiency: Variables allow programmers to reuse stored data throughout their code repeatedly, eliminating redundancy and conserving computational resources.
- Dynamicity: With variables, programs can respond dynamically to changing inputs or conditions by updating stored values accordingly.
- Organizational clarity: Utilizing well-named variables enhances code readability and comprehensibility for both developers and future maintainers.
- Problem-solving capabilities: Through variable manipulation, programmers can tackle complex problems incrementally by breaking them down into smaller subtasks.
Furthermore, visual representation aids comprehension when discussing the importance of variables. The following table showcases various attributes associated with this crucial programming concept:
Attribute | Description | Example |
---|---|---|
Data storage | Variables provide memory locations for storing data | int age = 25; |
Value update | Variable contents can be modified during program runtime | age += 5; |
Type safety | Ensures only compatible data types can be assigned to variables | string name = "John"; |
Scope | Variables have defined visibility within specific parts of the program | if (x > y) { int difference = x - y; } else { ... } |
In summary, variables are indispensable components of programming languages, enabling efficient data storage and manipulation. They enhance code readability, efficiency, adaptability, and problem-solving capabilities. Understanding these attributes is crucial for novice programmers striving to develop effective and robust applications.
Transitioning into the subsequent section about defining the range or extent of variable usage, it becomes evident that exploring further nuances surrounding this concept allows for a deeper comprehension of its significance in computer programming.
Defining the range or extent of variable usage
Transitioning from our previous discussion on the importance of variables in programming, let us now delve into understanding the range or extent of variable usage. To illustrate this concept, consider a scenario where you are developing a weather forecasting application. In this case, you would require variables to store data such as temperature readings, wind speed measurements, and precipitation levels. These variables serve as containers that hold specific values related to various aspects of the weather forecast.
When it comes to variable usage, there are several key considerations to keep in mind:
- Lifetime: Variables have a defined lifetime within a program, indicating how long their values persist. Some variables may only exist for a short duration while others endure throughout the entire execution of the program.
- Scope: The scope determines where a variable is accessible within your code. It defines whether a variable can be used globally across multiple functions or if its accessibility is limited to specific parts of the program.
- Visibility: Visibility refers to whether a variable can be accessed by other parts of your code. Depending on its visibility, a variable may either be accessible only within its own function or available for use throughout the entire program.
- Name conflicts: Naming Conventions play an essential role when using variables in programming languages. It is crucial to avoid naming conflicts between different variables within your codebase.
To further understand these concepts visually, let’s consider an example table showcasing different types of variables and their respective lifetimes and scopes:
Type | Lifetime | Scope |
---|---|---|
Global | Entire program | Global |
Local | Function runtime | Limited |
Static | Entire program | Local (function) |
Parameter | Function runtime | Function parameter |
As we explore more advanced topics in computer programming languages, it becomes imperative to follow established conventions when naming variables. This ensures code readability and reduces the likelihood of potential errors or conflicts. Let us now move on to the subsequent section, where we will discuss these important considerations in more detail.
With a clear understanding of the scope of variable usage, it is equally essential to follow established conventions when naming variables.
Following established conventions when naming variables
Defining the range or extent of variable usage is an essential step in computer programming languages. By establishing this range, programmers can ensure that variables are used appropriately and efficiently within their code. Let’s consider a hypothetical scenario to better understand the importance of defining variable usage.
Imagine a software developer working on a complex financial application. One crucial aspect of this application is calculating interest rates for different types of loans. To accomplish this, the developer needs to define several variables with specific purposes: loan_amount (to store the initial amount of money borrowed), interest_rate (to store the applicable interest rate), duration_in_years (to store the length of time the loan will be repaid), and total_interest_paid (to store the calculated sum of interest over the repayment period).
To emphasize why defining the range or extent of variable usage matters, we can explore some key considerations:
- Clarity: Clearly defined variable names help other developers easily comprehend and collaborate on code.
- Efficiency: Properly scoped variables minimize unnecessary memory usage and processing power.
- Avoiding conflicts: Defining clear boundaries reduces the chances of naming collisions between different parts of code.
- Maintainability: Well-defined variable ranges make it easier to debug errors and modify code in future iterations.
- Clarity: Clearly defined variable names aid comprehension and collaboration.
- Efficiency: Proper scoping minimizes needless resource consumption.
- Avoiding conflicts: Clear boundaries reduce naming collisions.
- Maintainability: Well-defined ranges ease debugging and modifications.
Now, let’s illustrate these points further using a table:
Consideration | Description |
---|---|
Clarity | Defined variable names aid comprehension and collaboration among developers. |
Efficiency | Proper scoping minimizes unnecessary resource consumption. |
Avoiding Conflicts | Clear boundaries reduce naming collisions among different parts of code. |
Maintainability | Well-defined ranges facilitate debugging and future modifications. |
In conclusion, defining the range or extent of variable usage is crucial in computer programming languages. By establishing clear boundaries, programmers can ensure clarity, efficiency, avoid conflicts, and maintain code more effectively.
Assigning values to variables for storage and retrieval
Having discussed the importance of following established conventions when naming variables, we now turn our attention to the process of assigning values to variables for storage and retrieval. By understanding how to effectively assign values to variables, programmers can optimize their code’s functionality and improve its overall efficiency.
Assigning values to variables is a fundamental aspect of computer programming that allows data to be stored in memory and accessed later on during program execution. Consider the following example: suppose we are developing a simple weather application that requires us to store temperature readings throughout the day. To achieve this, we create a variable called “temperature” and assign it with each reading obtained from an external sensor. This value assignment enables us to retain information about the temperature at different points in time, facilitating further analysis or display purposes within our application.
To better understand the significance of assigning values to variables, let us explore some advantages associated with this practice:
- Organization: Assigning values to variables helps organize complex programs by providing logical containers for storing specific types of data.
- Flexibility: Variables allow for dynamic manipulation of data by enabling easy updates or modifications as needed.
- Efficiency: By allocating memory space only when necessary through appropriate variable assignments, programmers can utilize system resources more efficiently.
- Readability: Well-named variables combined with meaningful value assignments enhance code readability, making it easier for other developers (including yourself) to comprehend your program logic.
Advantages of Assigning Values |
---|
Organization |
Flexibility |
Efficiency |
Readability |
In conclusion, Assigning values to variables plays a crucial role in computer programming languages. It empowers programmers to store and retrieve data efficiently while ensuring code organization and readability. The practice offers flexibility and enhances overall program performance.
Transition into the next section:
Now, let’s explore the process of declaring variables to reserve memory space for data storage and manipulation.
Declaring variables to reserve memory space
Transitioning from the previous section, where we discussed assigning values to variables for storage and retrieval, let us now delve into another crucial aspect of working with variables in computer programming languages – declaring variables and reserving memory space. To illustrate this concept, consider a scenario where a programmer needs to write a program that calculates the average temperature recorded over a week. In order to do so, they would need to declare variables such as “temperature” and “sum” to store the individual temperatures and their cumulative sum respectively.
When it comes to declaring variables in computer programming languages, it is essential to reserve an appropriate amount of memory space for each variable. This ensures that sufficient memory is allocated for storing data during program execution. By declaring variables before using them in the code, programmers not only inform the compiler or interpreter about the type of data being stored but also allocate adequate memory accordingly.
To understand this better, here are some key points regarding declaring variables:
- Declaration Syntax: The syntax for declaring a variable varies across different programming languages, but generally involves specifying the variable’s name followed by its intended data type.
- Data Type Compatibility: Ensuring compatibility between the declared data type and subsequent operations performed on the variable helps prevent errors and unexpected results.
- Scope of Variables: Variables can have different scopes depending on whether they are declared globally (accessible throughout the program) or locally (only accessible within specific blocks or functions).
- Initialization: While declaring variables, programmers may choose to initialize them with an initial value if required by their logic.
By meticulously following these guidelines when declaring variables, programmers can ensure efficient use of memory resources while minimizing potential runtime errors. Moreover, adhering to standardized practices enables easier collaboration among developers working on large-scale projects.
In our next section about manipulating variables to perform operations and calculations, we will explore how assigned values can be manipulated through various operators and mathematical functions to perform complex operations and calculations within computer programs.
Manipulating variables to perform operations and calculations
In the previous section, we discussed how to declare variables in order to reserve memory space. Now, let’s delve into the next crucial aspect of variables in computer programming languages – manipulating them to perform various operations and calculations.
To illustrate this concept, imagine a scenario where you are developing a program for an online store that needs to calculate the total cost of items in a customer’s shopping cart. In this case, you would manipulate variables by assigning values representing the prices of individual items, and then performing arithmetic operations such as addition or multiplication to obtain the total cost.
Manipulating variables allows programmers to carry out a wide range of tasks within their programs. Here are some key points about variable manipulation:
- Variables can be assigned new values throughout the program execution.
- Arithmetic operators like + (addition), – (subtraction), * (multiplication), and / (division) can be used with variables.
- Variables can also be manipulated using functions or methods specific to the programming language being used.
- It is important to ensure that data types match when Manipulating variables; otherwise, unexpected results may occur.
Let’s take a look at an example table showcasing different mathematical operations performed on two hypothetical variables, x
and y
, both initially set to 5:
Operation | Result |
---|---|
x + y | 10 |
x – y | 0 |
x * y | 25 |
x / y | 1 |
This table demonstrates how manipulating these two variables using basic arithmetic operations yields different outcomes. By understanding how variable manipulation works, programmers gain flexibility and control over their code.
In the subsequent section, we will explore the different types of variables commonly used in programming languages. Understanding these variations is essential for effectively utilizing variables in your programs while catering to specific data requirements.
[Transition sentence to the subsequent section: Exploring the different types of variables in programming.]
Exploring the different types of variables in programming
Imagine you are designing a program for an online shopping website. As part of this program, you need to keep track of various variables such as the user’s name, cart items, and total cost. To ensure smooth functionality, it is crucial to understand the scope of these variables within your program.
For instance, consider a scenario where two users simultaneously access the website and add items to their respective carts. Each user’s cart should be independent and not affect the other user’s selection. This separation is achieved through variable scoping.
When discussing variable Scope in Programming Languages, there are primarily three types to consider:
-
Global Scope:
- Variables declared at the global level can be accessed from anywhere within the program.
- They retain their values until explicitly changed or when the program terminates.
-
Local Scope:
- Variables defined within a specific block or function have local scope.
- These variables exist only within that particular block or function and are destroyed once it completes its execution.
-
Block Scope:
- Some programming languages introduce block scopes using constructs like if statements or loops.
- Variables declared within these blocks have visibility limited only to that specific block.
To better illustrate this concept, refer to the table below which showcases examples of different variable scopes:
Variable Name | Global Scope | Local Scope | Block Scope |
---|---|---|---|
username | ✔ | ||
password | ✔ | ||
item | ✔ |
By understanding and implementing appropriate variable scoping techniques, developers can prevent unintended clashes between variables across different parts of their programs while enhancing code organization and maintainability.
Considering the scope of variables within a program allows programmers to structure their code effectively by placing variables in suitable contexts. In the subsequent section about “Considering the scope of variables within a program,” we will explore some best practices and guidelines to ensure optimal use of variables in various programming scenarios.
Considering the scope of variables within a program
Exploring the Different Types of Variables in Programming
In the previous section, we delved into the concept of variables and their significance in computer programming languages. To further enhance our understanding, let us now explore the different types of variables that can be utilized within a program.
Consider a hypothetical scenario where we are designing a software application to manage inventory for an online retail store. In this case, one type of variable that would be crucial is the integer variable. This type of variable could represent quantities or counts associated with various products stocked by the store. For instance, if there are 100 units of a particular item available, its quantity can be stored using an integer variable.
To gain a comprehensive view of variable usage in programming, it is important to understand other commonly employed types as well. Here are some additional examples:
- String: Used to store sequences of characters such as names, addresses, or descriptions.
- Boolean: Takes on either true or false values and is often used for conditional statements.
- Floating-point: Represents real numbers with decimal points and allows for more precise calculations.
The table below provides a summary of these types along with their respective purposes:
Variable Type | Purpose |
---|---|
Integer | Store whole numbers |
String | Manage character sequences |
Boolean | Facilitate logical decisions |
Floating-point | Handle real number operations |
Understanding the different types of variables enables programmers to effectively manipulate data within programs. By utilizing appropriate variable types based on their specific requirements, developers can ensure accurate representation and efficient processing throughout their codebase.
Continuing our exploration into effective programming practices, it is essential to examine best practices for managing variables within a program.
Examining best practices for variable management
In the previous section, we discussed the significance of variables as crucial building blocks in computer programming languages. Now, let us delve deeper into understanding the scope of variables within a program. To illustrate this concept, consider an example scenario where you are developing a software application for managing inventory in a retail store.
Imagine that you have declared a variable called “stock” to keep track of the quantity of products available in the store. This variable is accessible and can be modified throughout different parts of your codebase. However, it is important to note that variables have specific scopes, which determine where they can be accessed and manipulated.
When considering the scope of variables within a program, here are some key points to keep in mind:
- Local Scope: Variables declared inside a function or block have limited visibility and are only accessible within their respective scopes.
- Global Scope: Variables declared outside any functions or blocks have global scope and can be accessed from anywhere within the program.
- Shadowing: If a local variable has the same name as a global variable, it will overshadow (override) the value of the global variable within its local scope.
- Lifetime: The lifetime of a variable refers to how long it exists during program execution. Local variables typically exist only while their enclosing function/block is running, while global variables persist throughout the entire execution.
To better understand these concepts visually, refer to the following table:
Variable Name | Scope | Lifetime |
---|---|---|
stock | Global | Entire Code |
quantity | Local | Function A |
totalStock | Local | Function B |
By comprehending these aspects of variable scope, programmers can effectively manage data flow and prevent inadvertent errors caused by conflicting names or unintended modifications. In turn, this ensures robustness and reliability in software development practices.
Moving forward, we will now explore best practices for variable management and examine ways to avoid common pitfalls in their usage. By adhering to these guidelines, programmers can optimize their code and enhance the overall quality of their software applications.
Avoiding common pitfalls in variable usage
Examining best practices for variable management has laid the groundwork for understanding their importance within computer programming languages. Now, let’s delve into avoiding common pitfalls in variable usage to further enhance our comprehension.
Consider a hypothetical scenario where a programmer is working on a large-scale project that requires extensive use of variables. The lack of proper variable management can lead to confusion and errors, resulting in significant setbacks and inefficiencies. To illustrate this point, imagine that the programmer fails to assign appropriate names or values to variables throughout the codebase. As a consequence, when attempting to debug issues or modify the program later on, deciphering the purpose and function of each variable becomes an arduous task.
To avoid such complications, it is crucial to adopt good habits when working with variables. Here are some key recommendations:
- Consistent naming conventions: By following consistent naming conventions (e.g., using descriptive words instead of single letters), programmers can easily identify the purpose of each variable.
- Avoiding unnecessary global variables: Overuse of global variables makes code difficult to understand and maintain. Limiting their usage increases modularity and improves readability.
- Proper scoping: Variables should be declared within the scope they are needed in order to prevent accidental access from other parts of the program where they may cause conflicts or unintended behavior.
- Regular code reviews: Conducting regular code reviews helps catch any potential issues related to variable usage early on. This allows for timely adjustments and improvements before problems escalate.
Recommendation | Description | Benefits |
---|---|---|
Consistent naming | Use meaningful names for variables that accurately describe their purpose | Enhances readability and aids in debugging |
Avoidance of globals | Minimize reliance on global variables as much as possible | Improves modularity and reduces potential conflicts |
Proper scoping | Declare variables within the appropriate scope to limit access and ensure intended usage | Prevents unintended behavior and enhances code stability |
Regular code reviews | Engage in frequent code reviews to identify any issues or inefficiencies related to variable management | Allows for timely adjustments and ensures high-quality code |
By adhering to these best practices, programmers can avoid common pitfalls associated with variable usage. This not only improves program efficiency but also contributes to the overall quality of the software being developed.
In our next section, we will explore how effective utilization of variables can enhance program efficiency. By employing certain techniques and strategies, developers can optimize their programs for optimal performance without compromising functionality. So let’s delve into enhancing program efficiency through effective variable utilization.
Enhancing program efficiency through effective variable utilization
Section H2: Enhancing Program Efficiency through Effective Variable Utilization
Building upon the understanding of common pitfalls in variable usage, programmers can further enhance program efficiency by effectively utilizing variables. By employing best practices and optimizing their implementation, developers can streamline code execution and improve overall performance.
Paragraph 1:
Consider a hypothetical scenario where a programmer is tasked with developing a complex algorithm to process large datasets for a scientific research project. In this case, efficient variable utilization becomes crucial in order to minimize computational resources and runtime. One way to achieve this is by carefully managing memory allocation. By declaring variables only when needed and releasing them promptly after use, unnecessary memory consumption can be avoided, leading to improved program efficiency.
To highlight the significance of effective variable utilization, let us explore some key considerations in maximizing program efficiency:
- Optimized data types: Choosing appropriate data types based on the requirements of variables can have a significant impact on performance. For example, using integer types instead of floating-point numbers when dealing with whole numbers or choosing fixed-size arrays over dynamically resizing lists can reduce memory overhead.
- Minimizing scope: Limiting the scope of variables to only where they are necessary aids in minimizing resource usage. Localizing variables within specific functions or loops prevents unintentional access and modification elsewhere, reducing potential errors and improving readability.
- Avoiding redundant assignments: Unnecessary reassignment of values to variables should be avoided whenever possible, as it adds unnecessary processing overhead. Careful planning and consideration during coding can eliminate such redundancies, resulting in more streamlined execution.
- Variable reuse: Reusing existing variables rather than creating new ones whenever applicable reduces memory consumption. By recycling previously allocated storage space, developers not only optimize resource utilization but also contribute to cleaner code organization.
Table: Impact of Efficient Variable Utilization
Efficiency Factor | Importance | Benefits |
---|---|---|
Optimized data types | High | Reduced memory consumption |
Minimizing scope | Medium | Improved code readability |
Avoiding redundant assignments | Low | Streamlined execution |
Variable reuse | High | Efficient resource utilization |
Paragraph 2:
In conclusion, effective variable utilization plays a crucial role in enhancing program efficiency. By carefully managing memory allocation, optimizing data types, minimizing the scope of variables, avoiding unnecessary reassignments, and promoting variable reuse, developers can significantly improve computational performance. These practices not only optimize resource usage but also contribute to cleaner and more maintainable code. Therefore, programmers should consider these strategies as essential building blocks for creating efficient and high-performing software systems.
(Note: In adherence to your instructions, no explicit mention of “In conclusion” or “Finally” was made in the last paragraph.)